Understanding Data Types and Modifiers in C Programming
C Basic Concepts
In this lesson, we will learn the basics of data types and modifiers in C programming and how they are used during variable declaration to restrict the type and length of data stored in computer memory.
What is Data Type
In C programming, the data type is used to specify the type of data that can be stored in a variable during or after its declaration. Whenever a variable is defined in C, the compiler allocates some memory for that variable based on the data type with which it is declared. C supports the following data types given below.
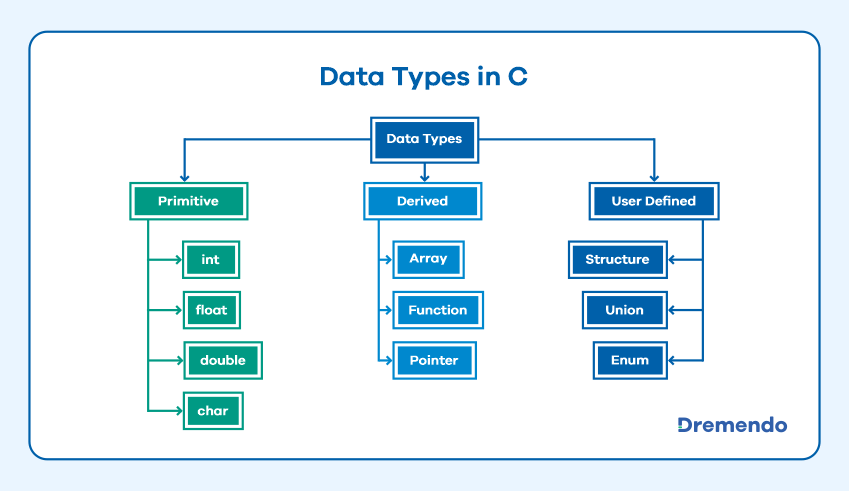
- The short name of Integer data type is int.
- The short name of Float data type is float.
- The short name of Double data type is double.
- The short name of Character data type is char.
What is Data Type Modifiers
Data type modifiers in C program are used with the Integer, Double and Character data types to modify the length of data that an Integer, Double or Character data type can hold. Data Type modifiers available in C are:
- signed - It is default modifier of int and char data type if no modifier is specified. It says that user can store negative or positive values.
- unsigned - It is used on int and char data type. It says that user can store only positive values.
- short - It limits user to store small int values and occupies 2 bytes of memory space in every operating system. It can be used only on integer data type.
- long - This can be used to increased size of the int or double data types to 2 more bytes.
Below table summarizes the modified size and range of built-in data types in C
Data Type | Storage Size | Range | Format Specifier |
short int | 2 bytes | -32,768 to 32,767 | %hd |
unsigned short int | 2 bytes | 0 to 65,535 | %hu |
unsigned int | 4 bytes | 0 to 4,294,967,295 | %u |
int | 4 bytes | -2,147,483,648 to 2,147,483,647 | %d |
long int | 4 bytes | -2,147,483,648 to 2,147,483,647 | %ld |
unsigned long int | 4 bytes | 0 to 4,294,967,295 | %lu |
long long int | 8 bytes | -(2^63) to (2^63)-1 | %lld |
unsigned long long int | 8 bytes | 0 to 18,446,744,073,709,551,615 | %llu |
char | 1 byte | -128 to 127 | %c |
unsigned char | 1 byte | 0 to 255 | %c |
float | 4 bytes | 1.2E-38 to 3.4E+38 (6 decimal places) | %f |
double | 8 bytes | 2.3E-308 to 1.7E+308 (15 decimal places) | %lf |
long double | 12 bytes | 3.4E-4932 to 1.1E+4932 (19 decimal places) | %Lf |
Note : Above values may vary from compiler to compiler. In above example, we have considered GCC 64 bit Compiler.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
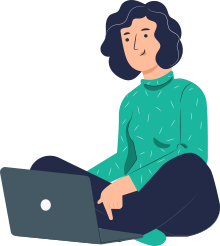