String in C Programming
String in C
In this lesson, we will understand what is String in C Programming along with some examples.
What is String in C
A String is a sequence of characters. In C programming, there is no specific data type to store String. So, we have to use Character Array to store a sequence of characters.
Declaration Syntax of a Character Array in C
char variable_name[size]
Here, size is the maximum number of characters that can be store in the character array.
Example
char a[15];
The above character array can store a string up to 15 characters long. You can use any size that fits your requirement.
Declaration and Initialization of a Character Array in C
In C programming a character array can be declared and initialized in 3 ways. Let's see all the three examples.
Example 1
char data[]= {'H','E','L','L','O','\0'};
In Example 1, we have declared a character array named data and initialize it with the string HELLO. We put a null character (‘\0’) at the end, which specifies the end of the string. So, the actual size of the array is 6, including the null character.
Example 2
char data[]= "HELLO";
In Example 2, we have used double quotes (") to initialize the word HELLO in the character array. Here we don’t have to specify the null character (‘\0’) because it is added automatically.
Thus, both the examples will look like as given in the picture below.
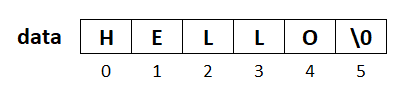
Example 3
char data[10]= "HELLO";
In Example 3, we have mentioned the size 10 and then use the quotes (") to initialize the word HELLO in the character array. In this case the array will look like as given in the picture below.

Store Characters in a Character Array
We can store character in a character array using 3 methods:
- Store characters one by one.
- Store characters using scanf() function.
- Store character using gets() function.
Let’s see all the examples one by one.
Example 1 (store characters one by one)
data[0]='H';
data[1]='E';
data[2]='L';
data[3]='L';
data[4]='O';
data[5]='\0';
Example 2 (store characters using scanf() function)
#include <stdio.h>
#include <conio.h>
int main()
{
char data[20];
char city[20];
printf("Enter Name ");
scanf("%[^\n]s",data);
fflush(stdin);
printf("Enter City ");
scanf("%[^\n]s",city);
return 0;
}
In example 2, we have used scanf() function to take input from the user. Notice that after taking the first input we have used fflush(stdin) function to clear the standard input.
If you don’t use the fflush(stdin) function, then enter key of the first input which is stored in the input buffer will be used as an input in the second array and you would not be able to input anything in the second array.
Example 3 (store characters using gets() function)
#include <stdio.h>
#include <conio.h>
int main()
{
char data[20];
char city[20];
printf("Enter Name ");
gets(data);
printf("Enter City ");
gets(city);
return 0;
}
In example 3, we have used gets() function to take input from the user. Here we don’t have to use fflush(stdin) function because gets() function always clear the input buffer after taking input.
Note: If you are taking int or float or double type input and after that, you are taking any character array input then use fflush(stdin) function before taking character array input. For example:
Example
#include <stdio.h>
#include <conio.h>
int main()
{
int roll;
float per;
char data[20];
char city[20];
printf("Enter Roll: ");
scanf("%d",&roll);
fflush(stdin); // clear the input buffer after numberic input
printf("Enter Name ");
gets(data);
printf("Enter Percentage % ");
scanf("%f",&per);
fflush(stdin); // clear the input buffer after numberic input
printf("Enter City ");
gets(city);
return 0;
}
Display Characters from a Character Array
To display all characters from a character array we can use either printf() or puts() function.
Example 1 (display characters using printf() function)
#include <stdio.h>
#include <conio.h>
int main()
{
char data[20];
char city[20];
printf("Enter Name ");
gets(data);
printf("Enter City ");
gets(city);
printf("Name: %s City: %s",data,city);
return 0;
}
Output
Enter Name Allen Smith Enter City California Name: Allen Smith City: California
Note: In the above example, inside printf() function we have used %s format specifier to print string on the screen.
Example 2 (display characters using puts() function)
#include <stdio.h>
#include <conio.h>
int main()
{
char data[20];
char city[20];
printf("Enter Name ");
gets(data);
printf("Enter City ");
gets(city);
printf("Name: ");
puts(data);
printf("City: ");
puts(city);
return 0;
}
Output
Enter Name Allen Smith Enter City California Name: Allen Smith City: California
Note: In the above example, the puts() function print the character array on the screen and then break the line automatically. Using puts() function, we cannot format our output. So if we want to format our output, then we have to use printf() instead of puts() function.
Access Individual Characters from a Character Array
We can access individual characters from a character array using for, while or do while loop.
Example
Program to input a word and print individual characters on separate lines.
#include <stdio.h>
#include <conio.h>
int main()
{
char data[20];
int i;
printf("Enter Name ");
gets(data);
for(i=0; data[i]!='\0'; i++)
{
printf("%c\n",data[i]);
}
return 0;
}
Output
Enter Name Dremendo D r e m e n d o
In the above example, we have run a 'for loop' from 0 until we get null (‘\0’) and then print the individual characters on the screen using printf() function on separate lines.
Two Dimensional Character Array in C
A Two Dimensional character array is used to store the list of strings. For example a list of country names, list of fruit names, etc.
Declaration Syntax of a Two Dimensional Character Array in C
char variable_name[row_size][column_size];
Here row_size is the number of rows we want to create in a 2D character array and, column_size is the number of maximum characters we want to store in each row.
Example
char list[5][10];
The above 2D character array named list can store 5 strings having maximum 10 characters in each string.
Declaration and Initialization of a Two Dimensional Character Array in C
In C programming a two dimensional character array can be declared and initialized in two ways. Let’s see both ways.
Example 1: (with row and column size mentioned)
char list[5][10]= {"Apple", "Banana", "Cherry"};
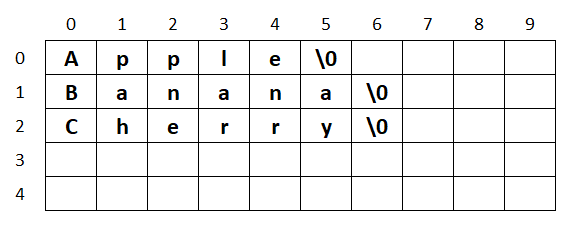
Example 2: (with only column size mentioned)
char list[][10]= {"Apple", "Banana", "Cherry"};
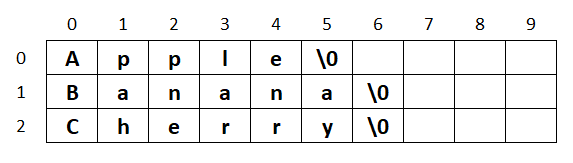
Store and Access Strings in a Two Dimensional Character Array
We can store and access string in 2D character array using for loop. Let’s see the example given below.
Example
Program to store and display the names of 3 fruits in a 2D character array.
#include <stdio.h>
#include <conio.h>
int main()
{
char list[3][10];
int r;
//store the name 3 fruits
printf("Enter 3 fruits name\n");
for(r=0; r<3; r++)
{
gets(list[r]);
}
//access the name of 3 fruits from the array
printf("\nNames of 3 fruits are\n");
for(r=0; r<3; r++)
{
printf("%s\n",list[r]);
}
return 0;
}
Output
Enter 3 fruits name Apple Banana Cherry Names of 3 fruits are Apple Banana Cherry
Note: To store and access the above 2D character array we just need to specify the array name along with the row index in square brackets [ ] as shown in the above example.