Typecasting in C Programming Language
C Basic Concepts
In this lesson, we will learn about what is Typecasting and different types of it. We will also go through some examples and take a quiz at the end.
What is Typecasting
In C program the process of changing one data type into another data type is known as Typecasting. There are two types of typecasting: Implicit and Explicit.
- Implicit Typecasting - This is also known as automatic typecasting. In this type of casting (conversion) the data is converted from one data type to another data type by the compiler itself without programmer interference.
- Explicit Typecasting - In this type of casting (conversion) the data is converted from one data type to another data type by the programmer itself.
Now let's see the examples for more understanding.
Example
int a = 5;
int b = 2;
float c = a/b; // result of c will be 2.0 and not 2.5 why?
Let me explain, as we have seen in the previous lessons that 3 data types can store numbers and they are int, float and double. As I have told you before that int is smaller than float and float is smaller than double in terms of storing capacity of numbers. Now see the explanation given below.
int value / int value = result will be an int value
float value / float value = result will be a float value
double value / double value = result will be a double value
int value / float value = result will be a float value
float value / int value = result will be a float value
int value / double value = it gives you result in double
double value / int value = it gives you result in double
float value / double value = result will be a double value
double value / float value = result will be a double value
Whenever an equation contains 2 or more different data types as shown in the above examples, the result of the equation will always be in a data type which is largest amongst other data types in the equation. That is because all other data types get automatically changed into the highest data type available in the equation and the final result of the equation will be in the highest data type. This type of conversion is known as Implicit Casting.
So what should we do to get the answer 2.5 of the equation c = a/b given above? Now let's see how we can achieve this.
float c = a/b;
I want answer 2.5 so I have to either convert variable a into float or variable b into float, to do so I have to change their data type from int to float inside the equation manually. This type of conversion is known as Explicit Casting. Now see the example below.
float c = (float)a / b;
Either you can change a or b into float. See the example.
float c = a / (float)b;
So, whenever you want to change the data type of a variable using explicit casting method, just write the name of the data type within ( ) before the variable name you want to convert, as shown in the example above.
Remember that changing the data type of the variable a or b using explicit casting method inside the equation does not change their original declared data type. It remains the same. The changes will be temporary for the sake of solving the equation only.
Now what will you do if the expression is like this:
float c = 10 / 7; // result of c will be 1.0
In that case, just change any of the two numbers into decimal number by adding a .0 to the end of the number like this:
float c = 10.0 / 7; // result of c will be 1.428571
Or like this:
float c = 10 / 7.0; // result of c will be 1.428571
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
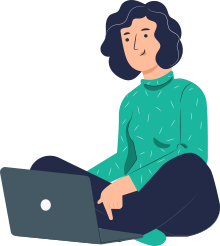