Operator Precedence in C Programming
Operators in C
In this lesson, we will learn what is the rule of Operator Precedence in C programming and how it works with some examples.
What is Operator Precedence
Operator Precedence in C programming is a rule that describe which operator is solved first in an expression. For example: * and / have same precedence and their associativity is Left to Right, so the expression 18 / 2 * 5 is treated as (18 / 2) * 5.
Let's take another example say x = 8 + 4 * 2; here value of x will be 16 and not 24 why, because * operator has a higher precedence than + operator. So 4*2 gets multiplied first and then adds into 8.
Operator Associativity means how operators of the same precedence are evaluated in an expression. Associativity can be either from left to right or right to left.
The table below shows all the operators in C with precedence and associativity.
Precedence and Associativity of C Operators
OPERATOR | DESCRIPTION | ASSOCIATIVITY |
( ) [ ] . -> ++ -- |
Parentheses Array Subscript Member Selector Post-Increment / Decrement |
left to right |
++ -- + - ! ~ (type) * & sizeof |
Pre-Increment / Decrement Unary plus / minus not operator and bitwise complement type casting Dereference operator Address of operator Determine size in bytes |
right to left |
* / % | Multiplication, Division and Modulus | left to right |
+ - | Addition and Subtraction | left to right |
<< >> | Bitwise leftshift and right shift | left to right |
< <= > >= |
relational less than / less than or equal to relational greater than / greater than or equal to |
left to right |
== != | Relational equal to / not equal to | left to right |
& | Bitwise AND | left to right |
^ | Bitwise exclusive OR | left to right |
| | Bitwise inclusive OR | left to right |
&& | Logical AND | left to right |
|| | Logical OR | left to right |
? : | Ternary operator | right to left |
= += -= *= /= %= &= ^= |= <<= >>= |
Assignment operator Addition / subtraction assignment Multiplication / division assignment Modulus and bitwise assignment Bitwise exclusive / inclusive OR assignment Bitwise leftshift / rightshift assignment |
right to left |
, | comma operator | right to left |
Please don't get confused after seeing the above table. They all are used in a different situation but not all at the same time. For solving basic equation we will consider the following operator precedence only.
- ( ) Brackets will be solved first.
- * / % Which ever come first from left to right in your equation.
- + - Which ever come first from left to right in your equation.
Now let's see some examples for more understanding.
Example 1
45 % 2 + 3 * 2 45 % 2 + 3 * 2 will be solved as per operator precedence rule 1 + 3 * 2 will be solved as per operator precedence rule 1 + 6 will be solved as per operator precedence rule 7 (Answer)
Example 2
19 + (5 / 2) + 4 % 2 - 6 * 3 19 + (5 / 2) + 4 % 2 - 6 * 3 will be solved as per operator precedence rule 19 + 2 + 4 % 2 - 6 * 3 will be solved as per operator precedence rule 19 + 2 + 0 - 6 * 3 will be solved as per operator precedence rule 19 + 2 + 0 - 18 will be solved as per operator precedence rule 21 + 0 - 18 will be solved as per operator precedence rule 21 - 18 will be solved as per operator precedence rule 3 (Answer)
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
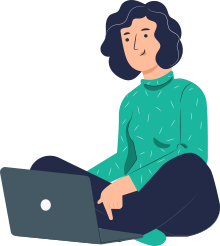