Assignment Operators in C++ Programming
Operators in C++
In this lesson, we are going to look at what is assignment operator and will learn the different types of assignment operator with the help of examples.
What is Assignment Operator
Assignment Operator in C++ is used for assigning value to a variable. Using the assignment operator we can assign the value which is on the right side of the operator to the variable on the left side of the operator.
Now let's see the examples for more understanding.
Example 1 (Assign Operator =)
int a, b;
a=5; // value 5 is assigned to variable a
b=a; // value of variable a which is 5 is assigned to the variable b
The = operator is used to assign the value from right to left. As you can see the value 5 which is on the right side of the operator = has been assigned to the left in the variable a. In the same way value of the variable a (5) which is on the right side of the operator = has been assigned to the left in the variable b
Note: The lines started with // are called comments which are used to provide information about lines of code. We will learn more about comments in our upcoming lessons.
Example 2 (Add and Assign Operator +=)
int a=2, b=10, c=5;
a+=2; // can be written as a=a+2 and result is 4
b+=c; // can be written as b=b+c and result is 15
The += operator is a combination of + and = operators. This operator first adds the current value of the variable on right to the value on the left and then assigns the result to the variable on the left.
Example 3 (Subtract and Assign Operator -=)
int a=6, b=10, c=5;
a-=2; // can be written as a=a-2 and result is 4
b-=c; // can be written as b=b-c and result is 5
The -= operator is a combination of - and = operators. This operator first subtract the current value of the variable on right from the value on the left and then assigns the result to the variable on the left.
Example 4 (Multiply and Assign Operator *=)
int a=7, b=10, c=5;
a*=2; // can be written as a=a*2 and result is 14
b*=c; // can be written as b=b*c and result is 50
The *= operator is a combination of * and = operators. This operator first multiplies the current value of the variable on left with the value on the right and then assigns the result to the variable on the left.
Example 5 (Divide and Assign Operator /=)
int a=18, b=10, c=5;
a/=2; // can be written as a=a/2 and result is 9 (quotient)
b/=c; // can be written as b=b/c and result is 2 (quotient)
The /= operator is a combination of / and = operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result (quotient) to the variable on the left.
Example 6 (Modulus and Assign Operator %=)
int a=6, b=10, c=8;
a%=2; // can be written as a=a%2 and result is 0 (remainder)
b%=c; // can be written as b=b%c and result is 2 (remainder)
The %= operator is a combination of % and = operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result (remainder) to the variable on the left.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
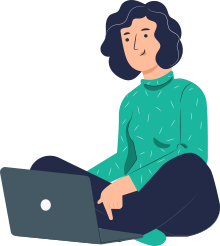