Dynamic Memory Allocation in C++ Programming
Pointer and Structure in C++
In this lesson, we will understand what is Dynamic Memory Allocation in C++ Programming along with some examples.
What is Dynamic Memory Allocation in C++
The process of allocating memory during runtime (execution of the program) is known as Dynamic Memory Allocation.
In C++ programming, the allocating and releasing of memory space is done, with the use of new and delete operator.
Let's see how to use these operators in C++ program to allocate and release the memory during runtime.
new Operator
The new operator is used to allocate space in the memory during runtime (execution of the program). This operator allocates a memory space of specified datatype and return the starting address to pointer variable.
Syntax of new Operator
pointer_variable = new datatype;
Example 1
#include <iostream>
#include <conio.h>
using namespace std;
int main()
{
int *ptr;
ptr=new int;
return 0;
}
Here ptr is an integer type pointer variable that holds the memory address allocated by new operator to store an integer type value.
Example 2
#include <iostream>
#include <conio.h>
using namespace std;
int main()
{
int *ptr;
ptr=new int[5];
return 0;
}
Here we allocate the memory space for 5 integers, and the base address is stored in the pointer variable ptr. See the example image given below.
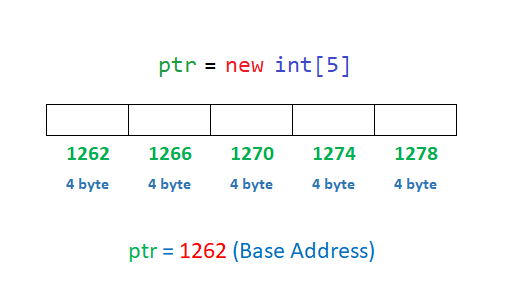
Example 3
C++ program to store and display 5 integer numbers by dynamic memory allocation using new operator.
#include <iostream>
#include <conio.h>
using namespace std;
int main()
{
int *ptr,i;
ptr=new int[5];
cout<<"Enter 5 numbers\n";
for(i=0; i<5; i++)
{
cin>>ptr[i];
}
for(i=0; i<5; i++)
{
cout<<ptr[i]<<" ";
}
return 0;
}
Output
Enter 5 numbers 12 89 47 64 23 12 89 47 64 23
In the above program, we are accessing the consecutive memory addresses using the index number starting from 0. So, ptr[0] means the first address, ptr[1] means the second address and so on.
In order to access each address using its index number we have executed a for loop from 0 to 4.
delete Operator
The delete operator is used to release the memory, which is dynamically allocated by the new operator.
Syntax of delete Operator
delete pointer_variable;
Example
#include <iostream>
#include <conio.h>
using namespace std;
int main()
{
int *a, *b;
// Allocate memory to store 1 integer
a=new int;
// Allocate memory to store 2 integers
b=new int[2];
// Release the memory allocated in pointer a
delete a;
// Release the all memories allocated in pointer b
delete []b;
return 0;
}
Note: For efficient use of memory, it is important to release the allocated space using the delete operator when the allocated space is no more required in the program.