Learn the Basic Structure of a C++ Program
Input and Output in C++
In this lesson, we will learn about the essential components of a C++ program and its basic structure.
Basic Structure of a C++ Program
Before we start learning how to write a program in C++, let us first understand what a basic structure of a C++ program looks like.
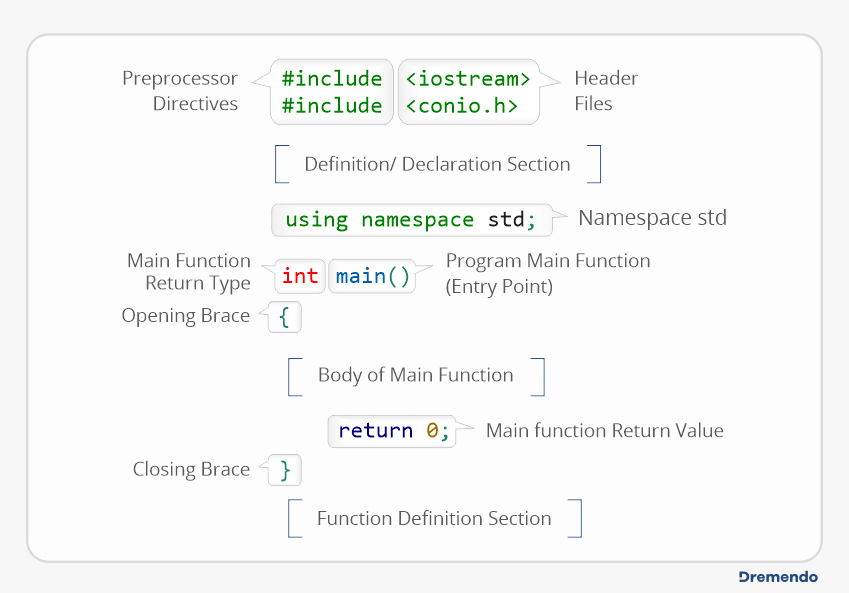
Example
This is how a simple basic structure of a C++ program looks like.
#include <iostream>
#include <conio.h>
using namespace std;
int main()
{
return 0;
}
Now we will discuss the various parts of the basic structure of a C++ program given above.
Preprocessor Directive
The Preprocessor Directive begins with the character #. It is used to include the necessary header file in a C++ program before compilation.
Header File
The Header File contains the function declaration and macro definition for C++ in-built library functions which we use in our C++ program during programing. When we include header file in C++ program using #include <filename.h> command, all codes inside the header file is included in the C++ program and then the program is sent to the compiler for compilation.
Namespace std
When we use using namespace std into the C++ program, then it does not require to write std:: in front of standard commands throughout the code. Namespace std contains all the classes, objects and functions of the standard C++ library.
Definition/Declaration Section
This section is used to define macro, structure, class and global variables to be used in the programs, that means you can use these variables throughout the program.
Program Main Function (Entry Point)
In C++, the main function is treated as the entry point of the program, it has a return type (and in some cases accepts inputs via parameters). The main function is called by the operating system when the user runs the program.
Main Function Return Type
In the latest standard of C++, int is used as the return type of the main function. It means that the C++ program on its successful completion will return a value which is of type int. The default value of the return type is 0 if the program execution is normal.
Opening Brace
This is called the Opening Brace {. Whatever we will write inside the main function, we will write it after the Opening Brace.
Body of Main Function
In this section, we will write our C++ program, which will be executed by the main function after compilation.
Main Function Return Value
The return value for main is used to indicate how the program exited. If the program execution is normal, a 0 return value is used. Abnormal termination(errors, invalid inputs, segmentation faults, etc.) is usually terminated by a non-zero return.
Closing Brace
This is called the Closing Brace }. We use the Closing Brace at the end of the program.
Function Definition Section
When we want to define our function that fulfills a particular requirement, we can define them in this section.