Mathematical Functions in C++ Programming
Mathematical Functions
In this lesson, we will understand what is Mathematical Function, and with the help of examples, we will see how to use mathematical functions in the C++ program.
List of C++ Mathematical Functions
C++ Mathematical functions are predefined functions which accept values and return the result. To use mathematical functions, we have to include math.h or cmath.h header file in our program.
With the help of mathematical functions we can easily solve a complex equation in our program, for example, if we want to find the square root of a number, then we can use sqrt() mathematical function to find the square root of a given number.
Let's see all the important mathematical functions available in C++ in more details with examples.
abs() Function
The abs() function returns the absolute value of an integer number. Here Absolute value means number without negative sign. The absolute value of a number is always positive.
Syntax of abs() function
int abs(int num);
An abs() function takes input in integer data type and return the result in integer data type.
Example
C++ program to input an integer and print its absolute value.
#include <iostream>
#include <conio.h>
#include <cmath>
using namespace std;
int main()
{
int n,x;
cout<<"Enter an integer number ";
cin>>n;
x=abs(n);
cout<<"Absolute value of "<<n<<" is "<<x;
return 0;
}
Output
Enter an integer number -18 Absolute value of -18 is 18
Here you can see that we have input -18 and pass the value to the abs() function. The abs() function returned the result in the variable x after removing the negative sign from the number. Thus the final output is 18.
Note: In order to find the absolute value of a float or double data type number you can use fabs() function [Syntax: double fabs(double num)].
sqrt() Function
The sqrt() function returns the square root of a positive number. Remember that square root of a negative can not be calculated.
Syntax of sqrt() function
double sqrt(double num);
A sqrt() function takes input in double data type and return the result in double data type. You can also pass an integer data type number to the sqrt() function but the number will implicitly convert into double data type as shown in the example below.
Example
C++ program to input an integer and print its square root.
#include <iostream>
#include <conio.h>
#include <cmath>
using namespace std;
int main()
{
int n;
double x;
cout<<"Enter an integer number ";
cin>>n;
x=sqrt(n);
cout<<"Square root of "<<n<<" is "<<x;
return 0;
}
Output
Enter an integer number 25 Square root of 25 is 5
Here you can see that we have input 25 and pass the value to the sqrt() function. The sqrt() function returned the result in the variable x after calculating the square root. Thus the final output is 5.
ceil() Function
The ceil() function returns the nearest integer number greater than the number passed as argument.
Syntax of ceil() function
double ceil(double num);
A ceil() function takes input in double data type and return the result in double data type. You can also pass a float data type number to the ceil() function but the number will implicitly convert into double data type as shown in the example below.
Example
C++ program to input a floating point number and print its ceil value.
#include <iostream>
#include <conio.h>
#include <cmath>
using namespace std;
int main()
{
float n,x;
cout<<"Enter a floating point (decimal) number ";
cin>>n;
x=ceil(n);
cout<<"Ceil value of "<<n<<" is "<<x;
return 0;
}
Output
Enter a floating point (decimal) number 12.4 Ceil value of 12.4 is 13
Here you can see that we have input 12.4 and pass the value to the ceil() function. The ceil() function returned the result in the variable x after calculating the ceil value of variable n. Thus the final output is 13.
floor() Function
The floor() function returns the nearest integer number less than the number passed as argument.
Syntax of floor() function
double floor(double num);
A floor() function takes input in double data type and return the result in double data type. You can also pass a float data type number to the floor() function but the number will implicitly convert into double data type as shown in the example below.
Example
C++ program to input a floating point number and print its floor value.
#include <iostream>
#include <conio.h>
#include <cmath>
using namespace std;
int main()
{
float n,x;
cout<<"Enter a floating point (decimal) number ";
cin>>n;
x=floor(n);
cout<<"Floor value is "<<n<<" is "<<x;
return 0;
}
Output
Enter a floating point (decimal) number 12.4 Floor value of 12.4 is 12
Here you can see that we have input 12.4 and pass the value to the floor() function. The floor() function returned the result in the variable x after calculating the floor value of variable n. Thus the final output is 12.
fmod() Function
The fmod() function returns the remainder of the division of two double data type numbers.
Syntax of fmod() function
double fmod(double x, double y);
A fmod() function takes two input in double data type and return the result in double data type. You can also pass float data type numbers to the fmod() function but the numbers will implicitly convert into double data type as shown in the example below.
Example
C++ program to input two floating point numbers and print the remainder after dividing the numbers.
#include <iostream>
#include <conio.h>
#include <cmath>
using namespace std;
int main()
{
float a,b,c;
cout<<"Enter two floating point (decimal) numbers\n";
cin>>a>>b;
c=fmod(a,b);
cout<<"Remainder = "<<c;
return 0;
}
Output
Enter two floating point (decimal) numbers 5.26 3.17 Remainder = 2.09
Here you can see that we have input two floating point numbers 5.26 and 3.17 and pass the values to the fmod() function. The fmod() function returned the result in the variable c after calculating the remainder of the division. Thus the final output is 2.09.
pow() Function
The pow() function is used to computes the power of a number.
Syntax of pow() function
double pow(double x, double y);
In mathematics the power is written as xy.
A pow() function takes two input (first as base value and second as power value) in double data type and return the result in double data type. You can also pass an integer or float data type numbers to the pow() function but the numbers will implicitly convert into double data type as shown in the example below.
Example
C++ program to input two integer numbers and print the power.
#include <iostream>
#include <conio.h>
#include <cmath>
using namespace std;
int main()
{
int a,b;
double c;
cout<<"Enter two integer numbers\n";
cin>>a>>b;
c=pow(a,b);
cout<<"Power = "<<c;
return 0;
}
Output
Enter two integer numbers 5 2 Power = 25
Here you can see that we have input two integer numbers 5 and 2 and pass the values to the pow() function. The pow() function returned the result in the variable c after calculating the power. Thus the final output is 25.
All the above functions are most commonly used mathematical functions. Below is the list of other mathematical functions that you can use in your program as per requirement.
List of other mathematical functions
Function | Syntax | Description |
cos | double cos(double x) | The cos() function returns the cosine of x, where x is expressed in radians. The return value of cos() is in the range [-1, 1]. |
acos | double acos(double x) | The acos() function returns the arc cosine of x, which will be in the range [0, pi]. x should be between -1 and 1. Value of pi=3.14159265. |
cosh | double cosh(double x) | The function cosh() returns the hyperbolic cosine of x. |
sin | double sin(double x) | The function sin() returns the sine of x, where x is given in radians. The return value of sin() will be in the range [-1, 1]. |
asin | double asin(double x) | The asin() function returns the arc sine of x, which will be in the range [-pi/2, +pi/2]. x should be between -1 and 1. Value of pi=3.14159265. |
sinh | double sinh(double x) | The function sinh() returns the hyperbolic sine of x. |
tan | double tan(double x) | The tan() function returns the tangent of x, where x is given in radians. |
atan | double atan(double x) | The function atan() returns the arc tangent of x, which will be in the range [-pi/2, +pi/2]. Value of pi=3.14159265. |
atan2 | double atan2(double y, double x) | The atan2() function computes the arc tangent of y/x, using the signs of the arguments to compute the quadrant of the return value. |
tanh | double tanh(double x) | The function tanh() returns the hyperbolic tangent of x. |
exp | double exp(double x) | The exp() function returns e (2.7182818) raised to the xth power. |
log | double log(double x) | The function log() returns the natural (base e) logarithm of x. |
log10 | double log10(double x) | The log10() function returns the base 10 (or common) logarithm for x. |
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
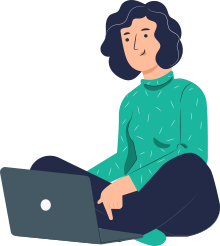