String Methods in C++ Programming
String in C++
In this lesson, we will learn about the different types of string methods in C++ programming and their uses with the help of examples.
C-Style Character String Methods in C++
The C-Style Character String methods are a collection of useful functions applied on a character array to solve various string related questions. Let's see all the important string methods one by one with examples.
strlen() Method
The strlen() method returns the length of the string without including null character ('\0'). The function is defined in <string.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <string.h>
using namespace std;
int main()
{
char s[30];
cout<<"Enter any string : ";
gets(s);
cout<<"Length of the string : "<<strlen(s);
return 0;
}
Output
Enter any string : Hello World Length of the string : 11
strcmp() Method
The strcmp() method compares the two strings and returns an integer value. If both the strings are same (equal) then this function would return 0 otherwise it may return a negative or positive value based on the comparison. The function is defined in <string.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <string.h>
using namespace std;
int main()
{
char str1[30]="Mango";
char str2[30]="Mango";
if(strcmp(str1,str2)==0)
{
cout<<"str1 and str2 both are same";
}
else
{
cout<<"str1 and str2 are not same";
}
return 0;
}
Output
str1 and str2 both are same
strcmpi() Method
The strcmpi() method compares the two strings without their case (upper and lower case) and returns an integer value. If both the strings are same (equal) then this function would return 0 otherwise it may return a negative or positive value based on the comparison. The function is defined in <string.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <string.h>
using namespace std;
int main()
{
char str1[30]="Mango";
char str2[30]="MaNgO";
if(strcmpi(str1,str2)==0)
{
cout<<"str1 and str2 both are same";
}
else
{
cout<<"str1 and str2 are not same";
}
return 0;
}
Output
str1 and str2 both are same
strcat() Method
The strcat() method concatenates two strings and returns the concatenated string. The function is defined in <string.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <string.h>
using namespace std;
int main()
{
char str1[10]="Hello";
char str2[10]="World";
strcat(str1,str2);
cout<<"String after concatenation: "<<str1;
return 0;
}
Output
String after concatenation: HelloWorld
strcpy() Method
The strcpy() method copies the string str2 into string str1, including null character ('\0'). The function is defined in <string.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <string.h>
using namespace std;
int main()
{
char str1[50]="string 1";
char str2[50]="string 2";
cout<<"str1 before copy: "<<str1<<endl;
/* this function has copied str2 into str1*/
strcpy(str1,str2);
cout<<"str1 after copy: "<<str1;
return 0;
}
Output
str1 before copy: string 1 str1 after copy: string 2
strupr() Method
The strupr() method converts a string to uppercase. The function is defined in <string.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <string.h>
using namespace std;
int main()
{
char str1[30]="hello world";
// convert the str1 into uppercase
strupr(str1);
cout<<"str1 is: "<<str1;
return 0;
}
Output
str1 is: HELLO WORLD
strlwr() Method
The strlwr() function converts a string to lowercase. The function is defined in <string.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <string.h>
using namespace std;
int main()
{
char str1[30]="HELLO WORLD";
// convert the str1 into lowercase
strlwr(str1);
cout<<"str1 is: "<<str1;
return 0;
}
Output
str1 is: hello world
strrev() Method
The strrev() method converts a string into reverse order. The function is defined in <string.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <string.h>
using namespace std;
int main()
{
char str1[30]="HELLO WORLD";
// convert the str1 into reverse order
strrev(str1);
cout<<"str1 is: "<<str1;
return 0;
}
Output
str1 is: DLROW OLLEH
tolower() Method
The tolower() method converts a character to lowercase. The function is defined in <ctype.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <ctype.h>
using namespace std;
int main()
{
char str1='H';
str1=tolower(str1);
cout<<"str1 is: "<<str1;
return 0;
}
Output
str1 is: h
toupper() Method
The toupper() method converts a character to uppercase. The function is defined in <ctype.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <ctype.h>
using namespace std;
int main()
{
char str1='h';
str1=toupper(str1);
cout<<"str1 is: "<<str1;
return 0;
}
Output
str1 is: H
isupper() Method
The isupper() method returns non-zero if the character is an uppercase letter. Otherwise, zero is returned. The function is defined in <ctype.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <ctype.h>
using namespace std;
int main()
{
char str1='A';
if(isupper(str1)!=0)
{
cout<<"str1 is in uppercase";
}
else
{
cout<<"str1 is not in uppercase";
}
return 0;
}
Output
str1 is in uppercase
islower() Method
The islower() method returns non-zero if the character is a lowercase letter. Otherwise, zero is returned. The function is defined in <ctype.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <ctype.h>
using namespace std;
int main()
{
char str1='a';
if(islower(str1)!=0)
{
cout<<"str1 is in lowercase";
}
else
{
cout<<"str1 is not in lowercase";
}
return 0;
}
Output
str1 is in lowercase
isalpha() Method
The isalpha() method returns non-zero if the character is an alphabet letter. Otherwise, zero is returned. The function is defined in <ctype.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <ctype.h>
using namespace std;
int main()
{
char str1='g';
if(isalpha(str1)!=0)
{
cout<<"str1 is an alphabet";
}
else
{
cout<<"str1 is not an alphabet";
}
return 0;
}
Output
str1 is an alphabet
isdigit() Method
The isdigit() method returns non-zero if the character is a digit between 0 and 9. Otherwise, zero is returned. The function is defined in <ctype.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <ctype.h>
using namespace std;
int main()
{
char str1='5';
if(isdigit(str1)!=0)
{
cout<<"str1 is a digit";
}
else
{
cout<<"str1 is not a digit";
}
return 0;
}
Output
str1 is a digit
ispunct() Method
The ispunct() method returns non-zero if the character is a printing character but not an alphabet, digit or a space. Otherwise, zero is returned. The function is defined in <ctype.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <ctype.h>
using namespace std;
int main()
{
char str1='?';
if(ispunct(str1)!=0)
{
cout<<"str1 is a punctuation";
}
else
{
cout<<"str1 is not a punctuation";
}
return 0;
}
Output
str1 is a punctuation
isspace() Method
The isspace() method returns non-zero if the character is some sort of space (i.e. single space, tab, vertical tab, form feed, carriage return, or newline). Otherwise, zero is returned. The function is defined in <ctype.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <ctype.h>
using namespace std;
int main()
{
char str1=' ';
if(isspace(str1)!=0)
{
cout<<"str1 is a space";
}
else
{
cout<<"str1 is not a space";
}
return 0;
}
Output
str1 is a space
atoi() Method
The atoi() method converts string into an integer, and returns that integer. The string should start with whitespace or some sort of number, and atoi() function will stop reading from string as soon as a non-numerical character has been read. The function is defined in <stdlib.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
using namespace std;
int main()
{
int x;
x=atoi("512");
cout<<"value of x = "<<x;
return 0;
}
Output
value of x = 512
atol() Method
The atol() method converts string into a long, then returns that value. The atol() function will read from string until it finds any character that should not be in a long. The resulting truncated value is then converted and returned. The function is defined in <stdlib.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
using namespace std;
int main()
{
long x;
x=atol("85642");
cout<<"value of x = "<<x;
return 0;
}
Output
value of x = 85642
atof() Method
The atof() method converts string into a double, then returns that value. The string must start with a valid number, but can be terminated with any non-numerical character, other than "E" or "e". The function is defined in <stdlib.h> header file.
Example
#include <iostream>
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
using namespace std;
int main()
{
double x;
x=atof("7462.3659");
cout<<"value of x = "<<x;
return 0;
}
Output
value of x = 7462.37
Standard String Class Methods in C++
The Standard String Class contains useful functions than can be applied on a string object to solve various string related questions. Let's see all the important standard string methods one by one with examples.
length() Method
The length() method returns the total number of characters (including space) present in the string object.
Example
#include <iostream>
#include <conio.h>
#include <string>
using namespace std;
int main()
{
string s="Dremendo";
cout<<"Length = "<<s.length();
return 0;
}
Output
Length = 8
at() Method
The at() method returns the character at the specified index. In a string, the first character starts from index 0.
Example
#include <iostream>
#include <conio.h>
#include <string>
using namespace std;
int main()
{
string s="Dremendo";
cout<<"Character at index 3 = "<<s.at(3);
return 0;
}
Output
Character at index 3 = m
insert() Method
The insert() method insert a specified string in the string object at the specified index.
Example
#include <iostream>
#include <conio.h>
#include <string>
using namespace std;
int main()
{
string s="Tier";
s.insert(2,"g");
cout<<"String = "<<s;
return 0;
}
Output
String = Tiger
erase() Method
The erase() method removes the specified number of characters from the string object including the specified index.
Example
#include <iostream>
#include <conio.h>
#include <string>
using namespace std;
int main()
{
string s="Hello World";
s.erase(5,6);
cout<<"String = "<<s;
return 0;
}
Output
String = Hello
append() Method
The append() method adds the specified character sequence at the end of the string object. We can also use the + operator to append character sequence at the end of the string object.
Example
#include <iostream>
#include <conio.h>
#include <string>
using namespace std;
int main()
{
string s="Hello";
s.append(" World");
cout<<"String = "<<s<<endl;
s=s+" I am learning C++ Programming";
cout<<"String = "<<s<<endl;
return 0;
}
Output
String = Hello World String = Hello World I am learning C++ Programming
compare() Method
The compare() method compares two strings lexicographically including case sensitive comparison. It returns a negative value if the first string is less then the second string. A positive value if the first string is greater than the second string. It returns zero if both are same.
Example
#include <iostream>
#include <conio.h>
#include <string>
using namespace std;
int main()
{
string s1="Superman";
string s2="Superman";
if(s1.compare(s2)==0)
{
cout<<"Both strings are same";
}
else
{
cout<<"Both strings are not same";
}
return 0;
}
Output
Both strings are same
substr() Method
The substr() method returns a new string which is a substring of the current string.
Example
#include <iostream>
#include <conio.h>
#include <string>
using namespace std;
int main()
{
string s="Superman";
cout<<"Print the 1st 3 characters of the current string : "<<s.substr(0,3)<<endl;
cout<<"Print the 1st 3 characters starting from index 2 : "<<s.substr(2,3)<<endl;
cout<<"Print all the characters starting from index 5 till end : "<<s.substr(5);
return 0;
}
Output
Print the 1st 3 characters of the current string : Sup Print the 1st 3 characters starting from index 2 : per Print all the characters starting from index 5 till end : man
find_first_of() Method
The find_first_of() method returns the index of the first occurrence of the specified character or string within the current string. The find_first_of() method accepts two arguments. The first argument is the string or character and the second optional argument is the index from where we want to check the occurrence. If the index is not given, it will start checking the occurrence from index 0. If the specified character or string is not present in the current string then it will return the npos value of the string.
Example
#include <iostream>
#include <conio.h>
#include <string>
using namespace std;
int main()
{
string s1="I am a boy and I love java programming.";
string s2="I";
if(s1.find_first_of("I")!=s1.npos)
{
cout<<"I found at index : "<<s1.find_first_of("I")<<endl;
}
if(s1.find_first_of("I",6)!=s1.npos)
{
cout<<"I found after index 6 at index : "<<s1.find_first_of("I",6)<<endl;
}
return 0;
}
Output
I found at index : 0 I found after index 6 at index : 15
find_last_of() Method
The find_last_of() method returns the index of the last occurrence of the specified character or string within the current string.
Example
#include <iostream>
#include <conio.h>
#include <string>
using namespace std;
int main()
{
string s1="I am a boy and I love java programming.";
string s2="I";
if(s1.find_last_of("I")!=s1.npos)
{
cout<<"Last I found at index : "<<s1.find_last_of("I")<<endl;
}
return 0;
}
Output
Last I found at index : 15
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
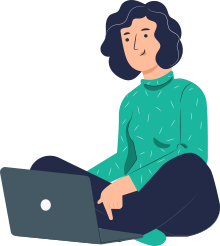