Comments in Java Programming
Input and Output in Java
In this lesson, we will understand how comments works in Java Programming with the help of examples.
What is Comments
In Java program Comments are portions of the code ignored by the compiler which allow the user to make simple notes in the relevant areas of the source code.
Comments in Java programming are used to provide information about lines of code. It is widely used for documenting code. There are 2 types of comments in Java programming.
- Single Line Comments - Single line comments starts with //.
- Multi-Line (Block) Comments - Multi-Line comments start with a slash asterisk /* and end with an asterisk slash */. It can occupy many lines of code.
Let's see some example for more understanding.
Example 1 (Single Line Comments)
public class Example
{
public static void main(String args[])
{
// Printing Hello World on the screen.
System.out.println("Hello World"); // Now we are going to print Hello World.
}
}
Output
Hello World
Here you can see that in line number 5 we have used a single line comments to explain what we are going to do on the next line. You can also write comment after the end of the statement as shown above in line number 6.
Example 2 (Multi-Line Comment)
public class Example
{
public static void main(String args[])
{
/* In this program we will learn how
to print Hello World on the screen */
System.out.println("Hello World");
//System.out.println("This is a Java tutorial");
}
}
Output
Hello World
Here you can see that from line number 5 to line number 6 we have used multi-line comments to explain what the program is all about. You can also comment on any statement that you don't want to execute in your program but want to keep it for future purpose as shown above in line number 8.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
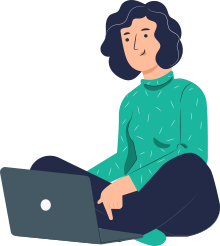