Float and Double Variables with Differences in Java
Java Basic Concepts
In this lesson, we will learn, what is Float and what is Double variable in Java programming. We will also look at some examples and will take a quiz at the end.
Float and Double Type Variables
As described before, an integer variable value has no fractional (decimal) part. Java uses two types of variables float and double that can store a decimal number. They each offer a different level of precision (ability to store numbers after the decimal point) as outlined below.
- float - A float data type variable can store about a maximum of 7 digits of precision. The memory capacity of the float data type is 4 bytes and can store a number between range -1.4e-045 to 3.4e+038.
- double - A double data type variable can store about a maximum of 15 digits of precision. The memory capacity of the double data type is 8 bytes and can store a number between range -4.9e-324 to 1.8e+308.
Note: The main difference between float and double data type is their memory capacity, precision and number range as outlined above.
Syntax of Declaring Float and Double Variable in Java
float variable_name;
double variable_name;
Here float is used for declaring Float data type and double is used for declaring Double data type. variable_name is the name of variable (you can use any name of your choice for example: a, b, c, alpha, etc.) and ; is used for line terminator (end of line).
Now let's see some examples for more understanding.
Example 1
Declare a float variable x and a double variable y
float x;
double y;
Example 2
Declare a float variable x to assign decimal number 18.286 and a double variable y to assign a decimal number 16722.02675585.
float x = 18.286f;
double y = 16722.02675585;
Note: Here f is used to denote a float type value. If no f is used in a decimal number, then the number is treated as double type value.
Example 3
Declare 3 float variables a, b, c and 3 double variable x, y, z
float a, b, c;
double x, y, z;
Example 4
Declare 3 float variables a, b and c to assign the float value 41.23, 87.335 and 62.471 respectively after that declare 3 float variables x, y and z to assign the double value 19.2347, 22.20 and 54.458691027 respectively
float a=41.23f, b=87.335f, c=62.471f;
double x=19.2347, y=22.20, z=54.458691027;
Example 5
Declare a float variable x and double variable y and assign the float value 12.365 and double value 79.2358 in the separate line respectively.
float x;
double y;
x = 12.365f;
y = 79.2358;
Example 6
Declare a float variable x and double variable y and assign the float value 7.18 and double value 11.62254 after that change their values to 44.23 and 67.48612 respectively.
float x=7.18f;
double y=11.62254;
x = 44.23f; // now the new value of x is 44.23
y = 67.48612; // now the new value of y is 67.48612
Note: If you want to store an integer number in a float or double type variable then you can do so as because int is smaller than float and float is smaller than double in terms of storing capacity of numbers. So an integer value can be stored in float type variable and a float value can be stored in a double type variable. Let's see the example given below.
float x=58;
double y=76;
The integer value 58 will be converted into float data type value and will be stored as 58.0. Similarly the integer value 76 will be converted into double data type value and will be stored as 76.0.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
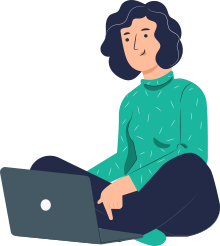