Packages in Java
Packages in Java
In this lesson, we will learn how to create and import a user defined package in java.
What is Package in Java?
In Java, a package is a directory (folder in windows) that contains classes, methods and interfaces. We create packages to store reusable classes, static methods and interfaces so that they can be used in another java program when required just by importing the package created earlier.
Advantages of Package in Java
- It helps to organize and group the reusable classes and interfaces in a directory so that we can easily maintain them.
- It provides access protection to classes and interfaces.
- It removes naming conflicts.
- It provides reusability of codes.
Types of Packages in Java
There are two types of packages available in Java, and they are:
- Built-in Packages
- User Defined Packages
Built-in Packages
Built-in packages are predefined packages that come with the JDK (Java Development Kit). There are many built-in packages in Java. Below is the list of some packages that are frequently used in Java programs are:
- java.lang
- java.io
- java.awt
- java.math
- java.util
- java.sql
- java.security
User Defined Packages
A user-defined package in Java is a package that a user creates to store reusable classes, static methods, and interfaces. A user-defined package is not part of the Java built-in packages.
By creating user-defined packages, developers can organize their code logically and meaningfully and prevent naming conflicts with other classes and interfaces. It also allows for better code reuse and maintenance in larger projects.
How to Create User Defined Package in Java
To create a user-defined package in Java, you need to follow these steps:
- Create a directory (folder) inside your java project folder with the name you want to use as a package name.
- Create a java file containing your Java class inside the directory you created earlier.
- Add the keyword package at the top of your Java source file, followed by the directory name that contains your source file. See the example given below.
package directory_name;
Let's assume that we have a java project directory called PackageExample that contains our program file and other packages, as given below in the diagram.
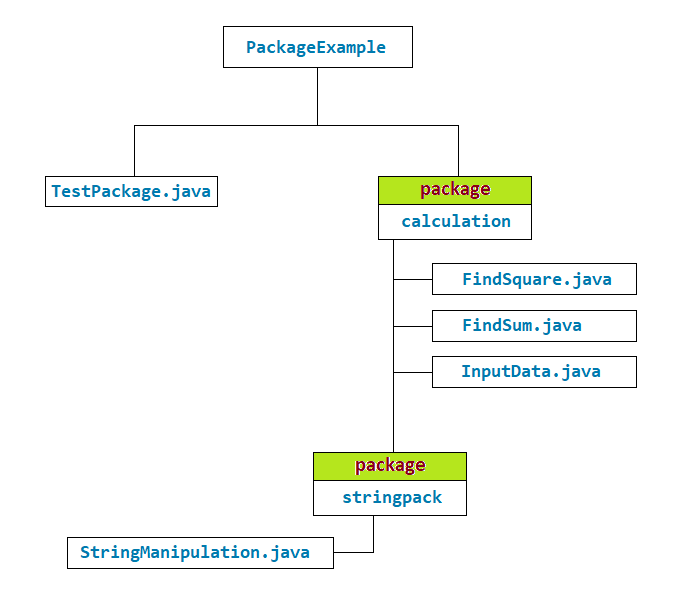
In the above image, TextPackage is the main program file that will show us how to import and use the user-defined package calculation and subpackage stringpack. Here calculation and stringpack are the name of directories (folders).
Let's see what code we have written in the source file created inside the package calculation and stringpack.
Code written in FindSquare.java file
package calculation;
public class FindSquare
{
public int square(int a)
{
return a*a;
}
}
Code written in FindSum.java file
package calculation;
public class FindSum
{
public int add(int a,int b)
{
return a+b;
}
}
Code written in InputData.java file
package calculation;
import java.util.Scanner;
public class InputData
{
private static Scanner sc=new Scanner(System.in);
public static int inputInt()
{
return sc.nextInt();
}
public static float inputFloat()
{
return sc.nextFloat();
}
public static char inputChar()
{
char c;
c=sc.nextLine().charAt(0);
return c;
}
}
Code written in StringManipulation.java file
package calculation.stringpack;
public class StringManipulation
{
public String joinString(String s1,String s2)
{
return s1+s2;
}
}
In the above source code, calculation is the name of main package and stringpack is the sub-package of main package calculation.
Code written in TestPackage.java file
// import all the classes directly present in the package calculation
import calculation.*;
// import the class StringManipulation present in the sub-package
// stringpack of the main package calculation
import calculation.stringpack.StringManipulation;
// import all the static methods of the class InputData
// present in the package calculation
import static calculation.InputData.*;
// import all the static methods of the class Math
// present in the package java.lang
import static java.lang.Math.*;
public class TestPackage
{
public static void main(String args[])
{
int x,y,z;
float a;
char c;
String data;
FindSum fs = new FindSum();
StringManipulation sm = new StringManipulation();
System.out.print("Enter a character ");
c=inputChar();
System.out.println("Enter 2 integers");
x=inputInt();
y=inputInt();
System.out.print("Enter a decimal number ");
a=inputFloat();
z=fs.add(x,y);
data=sm.joinString("Java"," Programming");
System.out.println("You have entered character: " + c);
System.out.println("Sum = "+z);
System.out.println(data);
System.out.println("Enter base and power");
System.out.println("Result = " + pow(inputInt(),inputInt()));
}
}
Output
Enter a character d Enter 2 integers 10 20 Enter a decimal number 18.412 You have entered character: d Sum = 30 Java Programming Enter base and power 2 3 Result = 8.0
Different Ways of Importing Packages in Java
We can import packages in a java program in different ways as required. Let's discuss each of the methods in detail.
Import a Single Class from a Package
To import a single class from a package, we need to write the import keyword followed by the package name, then give a dot and the name of the class we want to import into our program.
Example
import calculation.FindSquare;
In the above example, the calculation is the name of a user-defined package, and FindSquare is the name of a class present inside the package calculation.
Import all Classes from a Package
To import all classes from a package, we need to write the import keyword followed by the package name, then give a dot and an asterisk mark *.
Example
import calculation.*;
In the above example, the calculation is the name of a user-defined package, and * means we want to import all the classes directly present inside the package calculation but will not import the classes present in the sub-package of the package calculation.
Import a Single Class from a Sub-Package
To import a single class from a sub-package, we need to write the import keyword followed by the main package name, then give a dot and write the name of the sub-package with a dot and the name of the class we want to import in our program.
Example
import calculation.stringpack.StringManipulation;
The above example will import the class StringManipulation, which is present inside the sub-package stringpack of the main package calculation.
Import all Classes from a Sub-Package
To import all classes from a sub-package, we need to write the import keyword followed by the main package name, then give a dot and write the name of the sub-package with a dot and an asterisk mark *.
Example
import calculation.stringpack.*;
The above example will import all the classes which are present inside the sub-package stringpack of the main package calculation.
Import Static Members of a Class
In Java, the import static statement allows us to import the static members of a class, such as static fields and methods, and use them without specifying the class name. It can make your code more readable and concise.
Example
import static calculation.InputData.*;
import static java.lang.Math.*;
The above example will import all the static methods of the classes InputData and Math. Now we can use all the static methods of both the classes in our program without specifying their class name.
Example
// import all the static methods of the class InputData
// present in the package calculation
import static calculation.InputData.*;
// import all the static methods of the class Math
// present in the package java.lang
import static java.lang.Math.*;
public class TestPackage
{
public static void main(String args[])
{
System.out.println("Enter base and power");
System.out.println("Result = " + pow(inputInt(),inputInt()));
}
}
Output
Enter base and power 8 2 Result = 64.0