Integer Variable in Java Programming with Examples
Java Basic Concepts
In this lesson, we will learn about the Integer Variable in Java programming language. We will go through some examples of it and a quiz on it.
Integer Type Variable
In our earlier lesson we have learned about variable and how they are used to store values in computer memory. Now in this lesson we will learn how to declare variable in Java programming using an Integer Data Type.
So the first type of variable we need to know about is of type int - short for integer. This type of variable is used to store a whole number either positive or negative only (example: 10, 89, -24 etc). But if you try to store a fractional value (example: 12.36) in it then it will give loss of precision error in Java.
Syntax of Declaring Integer Variable in Java
int variable_name;
Here int is used for declaring Integer data type and variable_name is the name of variable (you can use any name of your choice for example: a, b, c, alpha, etc.) and ; is used for line terminator (end of line).
Now let's see some examples for more understanding.
Example 1
Declare an integer variable x.
int x;
Example 2
Declare an integer variable x to assign an integer value 10.
int x = 10;
Example 3
Declare 3 integer variables x, y and z in a single line.
int x, y, z;
Example 4
Declare 3 integer variables x, y and z to assign the integer value 42, 67 and 85 respectively in a single line.
int x=42, y=67, z=85;
Example 5
Declare an integer variable x and assign the integer value 18 in the second line.
int x;
x = 18;
Example 6
Declare an integer variable x and assign the integer value 76 and change its value to 54 in the next line.
int x = 76;
x = 54; // now the new value of x is 54
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
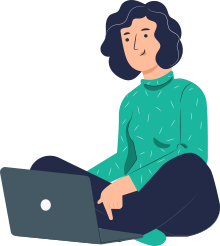