for Loop in Java Programming
Loops in Java
In this lesson, we will learn what is 'for' loop, and how many ways a 'for loop' can be used.
What is for Loop
A for loop in Java is a repetition control structure which allows us to write a loop that is executed a specific number of times. The for loop is also called an Entry Controlled loop because the test expression is tested before entering the loop body.
Syntax of for Loop
for(initialization expression; test expression; update expression)
{
// body of the loop
// statements we want to execute
}
Example
public class Example
{
public static void main(String args[])
{
int i;
for(i=1; i<=10; i=i+1)
{
System.out.println("Hello World");
}
}
}
Output
Hello World Hello World Hello World Hello World Hello World Hello World Hello World Hello World Hello World Hello World
In for loop, a loop variable is used to control the loop. First initialize this loop variable to some value, then check whether this variable is less than or greater than counter value. If statement is true, then loop body is executed and loop variable gets updated. Steps are repeated until test expression evaluates to false.
Let’s understand the above looping structure step by step.
- Initialization Expression: In the above example, int i=1; is our initialization expression. In this expression we have to initialize the loop variable to some value so that the loop will start from the initialized value. In the above example the loop will start from 1 and continues to execute until it crosses the value 10.
- Test Expression: In the above example, i<=10; is our test expression. The compiler will test the condition written in test expression. If the condition evaluates to true then the body of loop will execute and after that the program control will go to the update expression otherwise it will exit from the for loop.
- Update Expression: In the above example, i=i+1 is our update expression. After executing the body of the loop, the update expression increments the loop variable i by 1, and then move to test the condition written in test expression. If the condition evaluates to true, it will again execute the body of the loop and so on repeat the process until the test expression evaluates to false. When test expression evaluates to false, the loop terminates.
Let’s see some examples for more understanding.
Example 1
Java program to print the numbers from 1 to 10 on the screen using for loop.
public class Example
{
public static void main(String args[])
{
int i;
for(i=1; i<=10; i=i+1)
{
System.out.println(i);
}
}
}
Output
1 2 3 4 5 6 7 8 9 10
In the above example, we have run a for loop from 1 to 10. Each time when the loop runs, we print the value of the variable i on the screen on a separate line using System.out.println() statement. The loop ends when the value of i is more than 10.
Example 2
Java program to print all the even numbers from 10 to 20 on the screen using for loop.
public class Example
{
public static void main(String args[])
{
int i;
for(i=10; i<=20; i=i+2)
{
System.out.println(i);
}
}
}
Output
10 12 14 16 18 20
In the above example, we have run a for loop from 10 to 20. Each time when the loop runs, we print the value of the variable i on the screen on a separate line using System.out.println() statement and then increment the value of i by 2. The loop ends when the value of i is more than 20.
Example 3
Java program to print all the numbers from 10 to 1 in reverse order on the screen using for loop.
public class Example
{
public static void main(String args[])
{
int i;
for(i=10; i>=1; i=i-1)
{
System.out.println(i);
}
}
}
Output
10 9 8 7 6 5 4 3 2 1
In the above example, we have run a for loop from 10 to 1 in reverse order. Each time when the loop runs, we print the value of the variable i on the screen on a separate line using System.out.println() statement and then decrement the value of i by 1. The loop ends when the value of i is less than 1.
Example 4
Java program to input a 4-digit number and find the sum of its digits using for loop.
import java.util.Scanner;
public class Example
{
public static void main(String args[])
{
int i,n,r,sd=0;
Scanner sc=new Scanner(System.in);
System.out.print("Enter a 4 digit number ");
n=sc.nextInt();
if(n>=1000 && n<=9999)
{
for(i=n; i>0; i=i/10)
{
r=i%10; // return the last digit of the number as remainder
sd=sd+r;
}
System.out.println("Sum of digits = " + sd);
}
else
{
System.out.println("Not a 4 digit number");
}
}
}
Output
Enter a 4 digit number 2461 Sum of digits = 13
In the above example, we have input a 4-digit number. The loop starts with the 4-digit number and runs till the value of i is greater than 0. Each time when the loop runs, we find the last digit of the number using i%10 and add the digit in the variable sd and remove the last digit from the number using i=i/10. The loop ends when the value of i becomes 0 after removing all the digits from i.
Different ways of writing for loop
A for loop can be written in a various way depending on the requirement. Let’s see the different forms of writing a for loop.
Style 1
public class Example
{
public static void main(String args[])
{
int i;
for(i=1; i<=10; i=i+1)
{
System.out.println("Hello World");
}
}
}
Style 2
public class Example
{
public static void main(String args[])
{
int i=1;
for(; i<=10; i=i+1)
{
System.out.println("Hello World");
}
}
}
Style 3
public class Example
{
public static void main(String args[])
{
int i=1;
for(; i<=10;)
{
System.out.println("Hello World");
i=i+1;
}
}
}
Nested for Loop
Java programming allows using one for loop inside another for loop. This is known as nested for loop.
Syntax of for Loop
for(initialization expression; test expression; update expression)
{
// body of the outer loop
// statements we want to execute
for(initialization expression; test expression; update expression)
{
// body of the inner loop
// statements we want to execute
}
}
Example
public class Example
{
public static void main(String args[])
{
int i,j;
for(i=1; i<=5; i=i+1)
{
for(j=1; j<=i; j++)
{
System.out.print(j);
}
System.out.println();
}
}
}
Output
1 12 123 1234 12345
In the above example, we have run two loops, one outer loop and another inner loop. The outer loop runs from 1 to 5, and the inner loop runs from 1 to the current value of the outer loop (for example, when the value of i is 1, the inner loop runs from 1 to 1. When the value of i is 2, the inner loop runs from 1 to 2 and so on). The program ends when the outer loop test expression evaluates to false.
Infinite for Loop
Java infinite for loop is a loop that never ends because it does not have any test condition. It keeps on executing unless and until we terminate the loop using the break statement as shown in the example below.
Syntax of Infinite for Loop in Java
for(;;)
{
// body of the infinite for loop
}
Example
public class Example
{
public static void main(String args[])
{
int i=0;
for(;;)
{
System.out.println("Hello World");
i=i+1;
if(i==5)
{
break; // terminate the infinite loop when the value of i is 5
}
}
}
}
Output
Hello World Hello World Hello World Hello World Hello World
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
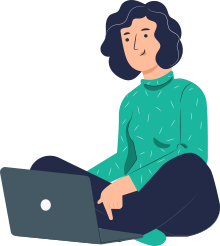