if else if Statement in Java Programming
Decision Making in Java
In this lesson, we will understand what is if else if statement and how to use it in Java program with the help of an example.
What is if else if Statement
Here, a user can decide among multiple options. The if statements in Java program are executed from the top down. As soon as one of the conditions controlling the if or else if is true, the statement associated with that if or else if is executed, and the rest of the else if is bypassed. If none of the conditions is true, then the final else statement will be executed.
if else if Statement Syntax
if(condition)
{
statement;
}
else if(condition)
{
statement;
}
else if(condition)
{
statement;
}
...
else
{
statement;
}
In the above syntax, you can see that we have started with if statement and then we are using else if statement. The ... means that we can write more else if statements if requires to check multiple options. If none of the conditions written inside if statements are true, then the final else statement will be executed. Here else statement is optional.
Now let's see an example for more understanding.
Example
Java program to check if an integer variable's value is equal to 10 or 15 or 20 using else if statement.
public class Example
{
public static void main(String args[])
{
int a=20;
if(a==10)
{
System.out.print("value of a is 10");
}
else if(a==15)
{
System.out.print("value of a is 15");
}
else if(a==20)
{
System.out.print("value of a is 20");
}
else
{
System.out.print("value of a is different");
}
}
}
Output
value of a is 20
Here you can see that the first condition (a==10) is false so the second condition (a==15) written in the else if statement is checked and the condition is also false, again the third condition (a==20) written in the else if statement is checked and this time the condition is true, so the statement which is written inside the curly braces { } of second else if statement has executed and the output is printed on the screen.
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
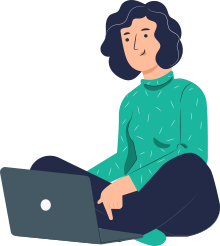