Learn the Basic Structure of a Java Program
Input and Output in Java
In this lesson, we will learn about the essential components of a Java program and its basic structure.
Basic Structure of a Java Program
In this tutorial, we will explore a Java program's basic structure and its components. By understanding the basics of a Java program, you will be better equipped to write your code and build more advanced programs.
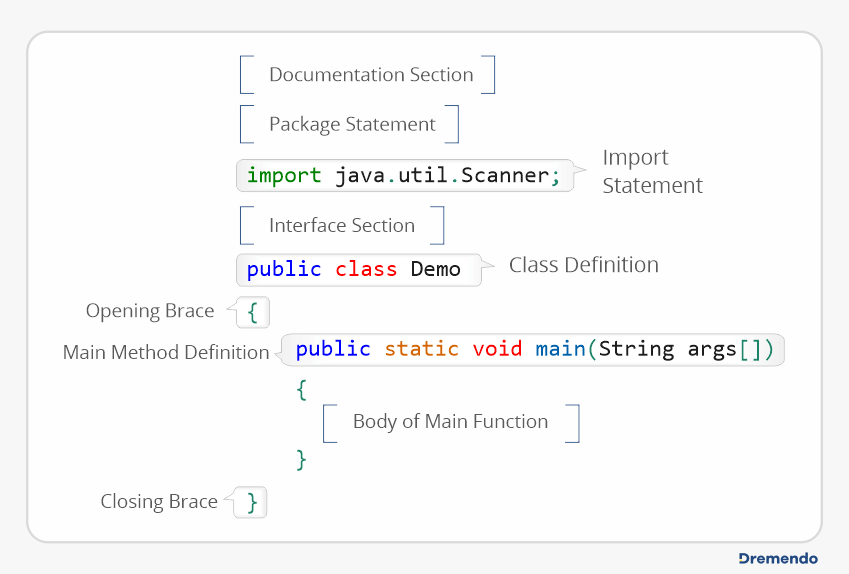
Example
This is how a simple basic structure of a Java program looks like.
public class Demo
{
public static void main(String args[])
{
}
}
Now we will discuss the various parts of the basic structure of a Java program given above.
Documentation Section
It is used to improve the readability of the program. It consists of comments in Java which include basic information such as the method’s usage or functionality to make it easier for the programmer to understand it while reviewing or debugging the code. This statement is optional.
Package Statement
There is a provision in Java that allows us to declare our classes in a collection called package. There can be only one package statement in a Java program and it has to be at the beginning of the code before any class or interface declaration. This statement is optional.
Import Statement
Many predefined classes are stored in packages in Java, an import statement is used to refer to the classes stored in other packages. An import statement is always written after the package statement but it has to be before any class declaration.
Interface Section
This section is used to specify an interface in Java. It is an optional section which is mainly used to implement Multiple Inheritance in Java. An interface is a lot similar to a class in Java but it contains only constants and method declarations.
Class Definition
A Java program may contain several class definitions, classes are an essential part of any Java program. Every program in Java will have at least one class with the main method. The class that contains the main method must be declared as public. For example:
- public class Test - This creates a class called Test. You should make sure that the class name starts with a capital letter, and the public word means it is accessible from any other classes.
Braces (Both Opening and Closing Brace)
The curly braces are used to group all the commands together. To make sure that the commands belong to a class or a method.
Main Method Definition
In Java, the main method is treated as the entry point of the program, The main method is called by the operating system when the user runs the program.
For Example: public static void main(String args[])
- public - When the main method is declared public, it means that it can be used outside of the declared class as well.
- static - The word static means that we want to access a method without making object of the class within which the method is declared. We call the main method without creating any objects.
- void - The word void indicates that it does not return any value. The main method is declared as void because it does not return any value.
- main - The main is the method, which is an essential part of any Java program.
- String args[] - It is an array where each element is a string, which is named as args. If you run the Java code through a console, you can pass the input parameter. The main() takes it as an input.