Types of Inheritance in Java Programming
OOPs in Java
In this lesson, we will understand how many types of Inheritance are their in Java and how to use them in class object programming.
Types of Inheritance in Java
There are four types of inheritance available in Java, and they are:
- Single Inheritance
- Multilevel Inheritance
- Multiple Inheritance
- Hierarchical Inheritance
Single Inheritance
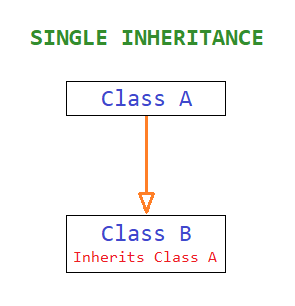
When a class inherits another class, this type of inheritance is called Single Inheritance. It is the most simple form of inheritance in Java.
The above image shows an example of single inheritance in which class B inherits class A. Thus class A can be called a base class or parent class, and class B can be called a child class or subclass or derived class.
Example of Single Inheritance
class ClassA
{
public void display()
{
System.out.println("In Class A");
}
}
class ClassB extends ClassA
{
public void display()
{
super.display();
System.out.println("In Class B");
}
}
public class Example
{
public static void main(String args[])
{
ClassB x= new ClassB();
x.display();
}
}
Output
In Class A In Class B
Multilevel Inheritance
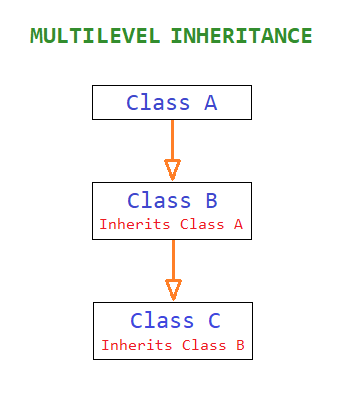
When a class is created by inheriting another derived class which is previously derived using another class, this type of inheritance is called Multilevel Inheritance.
In the above example, class C has been derived from class B, which is derived from class A.
Example of Multilevel Inheritance
class ClassA
{
public void display()
{
System.out.println("In Class A");
}
}
class ClassB extends ClassA
{
public void display()
{
super.display();
System.out.println("In Class B");
}
}
class ClassC extends ClassB
{
public void display()
{
super.display();
System.out.println("In Class C");
}
}
public class Example
{
public static void main(String args[])
{
ClassC x= new ClassC();
x.display();
}
}
Output
In Class A In Class B In Class C
Multiple Inheritance
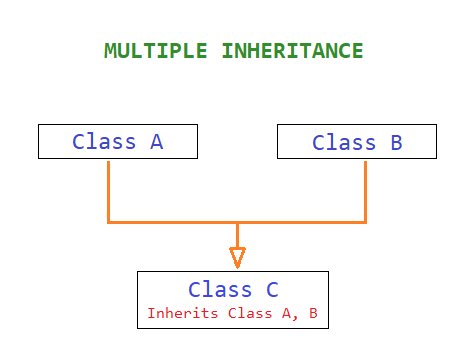
When a class is derived by inheriting two or more classes, this type of inheritance is called Multiple Inheritance.
This type of inheritance is not directly supported in Java and is achieved through the use of interfaces as show in the image below.
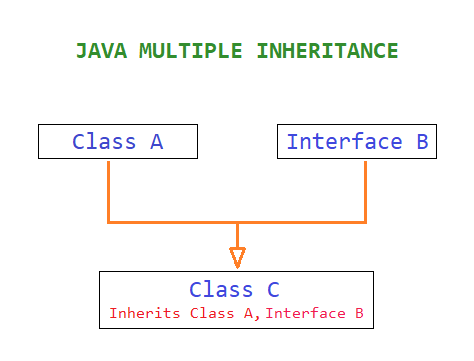
In the above image, class C has been derived by inheriting class A and interface B.
Example of Multiple Inheritance
class ClassA
{
public void display()
{
System.out.println("In Class A");
}
}
interface InterfaceB
{
public void show();
}
class ClassC extends ClassA implements InterfaceB
{
public void show()
{
System.out.println("Interface B");
}
public void display()
{
super.display();
System.out.println("In Class C");
}
}
public class Example
{
public static void main(String args[])
{
ClassC x= new ClassC();
x.show();
x.display();
}
}
Output
Interface B In Class A In Class C
Hierarchical Inheritance
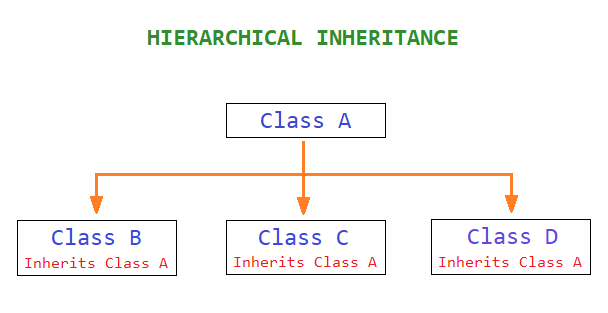
When two or more classes inherit the same base class, this type of inheritance is called Hierarchical Inheritance.
In the above image, classes B, C and D inherit the same base class A.
Example of Hierarchical Inheritance
class ClassA
{
public void display()
{
System.out.println("In Class A");
}
}
class ClassB extends ClassA
{
public void display()
{
super.display();
System.out.println("In Class B");
}
}
class ClassC extends ClassA
{
public void display()
{
super.display();
System.out.println("In Class C");
}
}
class ClassD extends ClassA
{
public void display()
{
super.display();
System.out.println("In Class D");
}
}
public class Example
{
public static void main(String args[])
{
ClassB x= new ClassB();
ClassC y= new ClassC();
ClassD z= new ClassD();
x.display();
y.display();
z.display();
}
}
Output
In Class A In Class B In Class A In Class C In Class A In Class D