switch case Statement in Java Programming
Decision Making in Java
In this lesson, we will understand what is switch case statement, and how it works in a Java Programming with the help of examples.
What is switch case Statement
In Java program a switch statement is used to compare an expression’s output value from a list of values, where each value is a case. When the expression’s output value is equal to one of the case’s value, then the statements following that case are executed.
A break statement ends the switch case. The optional default case is used when the value the test expression does not match with any of the case’s values.
switch Syntax
switch(expression)
{
case value:
statements;
break;
case value:
statements;
break;
...
...
// you can have any number of case statements.
default: // Optional
statements;
}
In the above syntax, we will write our test expression in place of the expression if the test expression's output value matches with any of the case's value then the statements following that case are executed. A break statement ends the switch case.
If text expression's output value does not match with any of the case's value then statements following the default (optional case) will execute. In default case, break is not required because it the last case after that the switch statement will ends automatically.
The following rules apply to switch case statements:
- The expression's output value statement must be of byte, short, char, int or string.
- You can have any number of case statements within a switch. Each case is followed by a value and a colon :.
- The value of a case must be a constant value and it must be of the same data type as the output value of the test expression in the switch statement.
- When the value being switched on is equal to a case's value, then the statements following that case will execute until a break statement is reached.
- Duplicate case values are not allowed.
- When a break statement is reached, the switch terminates, and the flow of control jumps to the next line following the switch statement.
- Every case needs to contain a break. If no break appears, the flow of control will fall through to subsequent cases until a break is reached.
- A switch statement can have an optional default case, which must appear at the end of the switch. The default case can be used for performing a task when none of the cases is true. No break is needed in the default case.
- Nesting of switch statements are allowed, which means you can have switch statements inside another switch. However nested switch statements should be avoided as it makes program more complex and less readable.
Now let's see some examples for more understanding.
Example 1
Java program to input any day number between 1 to 7 and print day name of the given number, example 1=Sunday, 2=Monday and so on.
import java.util.Scanner;
public class Example
{
public static void main(String args[])
{
int d;
Scanner sc=new Scanner(System.in);
System.out.print("Enter day number: ");
d=sc.nextInt();
switch(d)
{
case 1:
System.out.print("Sunday");
break;
case 2:
System.out.print("Monday");
break;
case 3:
System.out.print("Tuesday");
break;
case 4:
System.out.print("Wednesday");
break;
case 5:
System.out.print("Thursday");
break;
case 6:
System.out.print("Friday");
break;
case 7:
System.out.print("Saturday");
break;
default:
System.out.print("Invalid Number");
}
}
}
Output
Enter day number: 5 Thursday
Here you can see that we have input 5 as the value of d and the variable d is used as an expression in switch statement and its value matches with the value of the fifth case so the statement following the fifth case has executed and the output (Thursday) is printed on the screen.
Example 2
Java program to input a number and check if it is even or odd number.
import java.util.Scanner;
public class Example
{
public static void main(String args[])
{
int n;
Scanner sc=new Scanner(System.in);
System.out.print("Enter a number: ");
n=sc.nextInt();
switch(n%2)
{
case 0:
System.out.print("Even Number");
break;
case 1:
System.out.print("Odd Number");
break;
}
}
}
Output
Enter a number: 26 Even Number
Here you can see that we have input 26 as the value of n and the test expression of the switch statement is (n%2) so the output value of the test expression is 0 and it matches with the value of the first case so the statement following the first case has executed and the output (Even Number) is printed on the screen. Here the default case is not required as because the output of the text expression is either 0 or 1 only.
Example 3
Java program to print remark according to the grade obtained.
import java.util.Scanner;
public class Example
{
public static void main(String args[])
{
char g='B';
System.out.println("Grade:\t" + g);
switch(g)
{
case 'A':
System.out.print("Remark:\tExcellent!");
break;
case 'B':
case 'C':
System.out.print("Remark:\tWell Done");
break;
case 'D':
System.out.print("Remark:\tFail");
break;
default:
System.out.print("Invalid Grade");
}
}
}
Output
Grade: B Remark: Well Done
Here you can see that we have taken the grade B and it is matching with the case 'B' so the output (Well Done) is printed on the screen. You can also see that case 'B' and case 'C' has a common block of statement to execute. This is an example of Multi-Case statement where one or more case has a common block of statement to execute. You can see that we have used \t (escape sequence character) to print tab space on the screen.
Example 4 (Menu driven program using switch statement)
A switch statement is mainly useful in a menu based program where some menus will be printed on the screen and the user will choose an option from the given menu and the program associated with that menu will execute. Lets see an example for more understanding.
Java menu based program to find addition, subtraction, multiplication and division of to integer numbers.
import java.util.Scanner;
public class Example
{
public static void main(String args[])
{
int a,b,ch;
Scanner sc=new Scanner(System.in);
System.out.println("1 Addition");
System.out.println("2 Subtraction");
System.out.println("3 Multiplication");
System.out.println("4 Division");
System.out.print("Enter your choice: ");
ch = sc.nextInt();
switch(ch)
{
case 1:
System.out.println("Enter 2 integer numbers");
a=sc.nextInt();
b=sc.nextInt();
System.out.print("Addition=" + (a+b));
break;
case 2:
System.out.println("Enter 2 integer numbers");
a=sc.nextInt();
b=sc.nextInt();
System.out.print("Subtraction=" + (a-b));
break;
case 3:
System.out.println("Enter 2 integer numbers");
a=sc.nextInt();
b=sc.nextInt();
System.out.print("Multiplication=" + (a*b));
break;
case 4:
System.out.println("Enter 2 integer numbers");
a=sc.nextInt();
b=sc.nextInt();
System.out.print("Division=" + (a/b));
break;
default:
System.out.print("Invalid Choice");
}
}
}
Output
1 Addition 2 Subtraction 3 Multiplication 4 Division Enter your choice: 3 Enter 2 integer numbers 5 2 Multiplication=10
Here you can see that we have printed the menu on the screen and based on the option selected by the user the matching case executes.
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
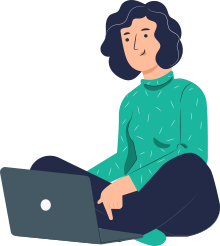