Function in Java Programming
Function in Java
In this lesson, we will understand what is Function in Java Programming and how to create them along with some examples.
What is Function in Java
A Function in Java is a block of statements that is defined to perform a specific task. A function is also called a reusable code because once it is created, it can be used again and again.
To clearly understand a function let's take a real life example of a function.
In the early days, we used to wash our clothes manually by using our hands, and we used to repeat this task daily. But nowadays, we wash our clothes using a washing machine that does the same task that we used to do in our early days by hands. Here, the washing machine is a perfect example of a function, we can use it, again and again, to wash our clothes whenever required.
Function Categories
In Java, there are two categories of functions available, and they are:
- Built-in Functions : These functions are predefined, and we can use them any time we want in our Java program. For example pow(), sqrt(), min(), etc.
- User Defined Functions : These functions are defined or created by the programmer for performing a specific task in a program.
Note: In this lesson, we will learn how to create and use a user-defined function in Java.
Types of User Defined Function
The user-defined function can be of two types and they are:
- Void Function
- Return Type Function
Void Function
This type of function does not return any value or result to the caller program (program that calls the function). To create void function we have to used the keyword void as per the syntax given below.
Syntax of Creating a Void Function
public static void function_name(parameters)
{
statement 1;
statement 2;
...
}
Example 1 (without parameters)
public static void message()
{
System.out.println("Hello I am learning how to create void function in Java");
}
Example 2 (with parameters)
public static void sum(int a, int b)
{
int c;
c=a+b;
System.out.println("Sum of " + a + " and " + b + " is " + c);
}
Note: Parameters are also called Arguments. Function can be created with or without parameters depending on the requirements.
Calling a Void Function
To call a void function, we have to call it by its name. See the complete example given below.
Example
public class Example
{
public static void message()
{
System.out.println("Hello I am learning how to create void function in Java.");
}
public static void sum(int a, int b)
{
int c;
c=a+b;
System.out.println("Sum of " + a + " and " + b + " is " + c);
}
public static void main(String args[])
{
message();
sum(10,20);
}
}
Output
Hello I am learning how to create void function in Java. Sum of 10 and 20 is 30
In the above program, we have declared two void functions message() and sum() before the main() function. The message() function does not accept any argument but displays a text on the screen whenever we call it. On the other hand, the sum() function accept two arguments and display their sum on the screen.
We have called both the functions from within the main() function. So, main() function is known as caller function and message() and sum() are known as called function because they are called by the main() function to process the task.
Return Type Function
This type of function returns the value or result to the caller program (the program that calls the function). The function return type can be int, float, double, char or any other type depends on the requirement.
Syntax of Creating a Return Type Function
public static return_type function_name(parameters)
{
statement 1;
statement 2;
...
return result:
}
Example 1 (without parameters)
public static float pi()
{
return 3.142f;
}
Example 2 (with parameters)
public static int sum(int a, int b)
{
int c;
c=a+b;
return c;
}
In the above example, the sum() function returns the value of c whose data type is int. So the return type of sum() function is int. The return type of a function depends upon the return type of the final result. If the final result is float type then the return type of the function must be float.
Calling a Return Type Function
We can call a return type function just like we call a void function. See the complete example given below.
Example
public class Example
{
public static float pi()
{
return 3.142f;
}
public static int sum(int a, int b)
{
int c;
c=a+b;
return c;
}
public static void main(String args[])
{
int x=10, y=20, z;
System.out.println("The value of PI is 22/7 = " + pi());
z=sum(x,y);
System.out.println("Sum of " + x + " and " + y + " is " + z);
}
}
Output
The value of PI is 22/7 = 3.142 Sum of 10 and 20 is 30
In the above program, we have declared two return type functions pi() and sum() before the main() function. The pi() function does not accept any argument but returns the value 3.142 to the caller program (main function).
The sum() function accept two arguments, we pass the values of x and y to the sum() function. The variable a and b of the sum() function received the values from x and y and returns their sum to the caller program (main function) after calculation.
Flow of Execution of Function
The Flow of Execution of Function refers to the order in which statements are executed in the main program when any function is called. Let's see the execution of the above program using a diagram.
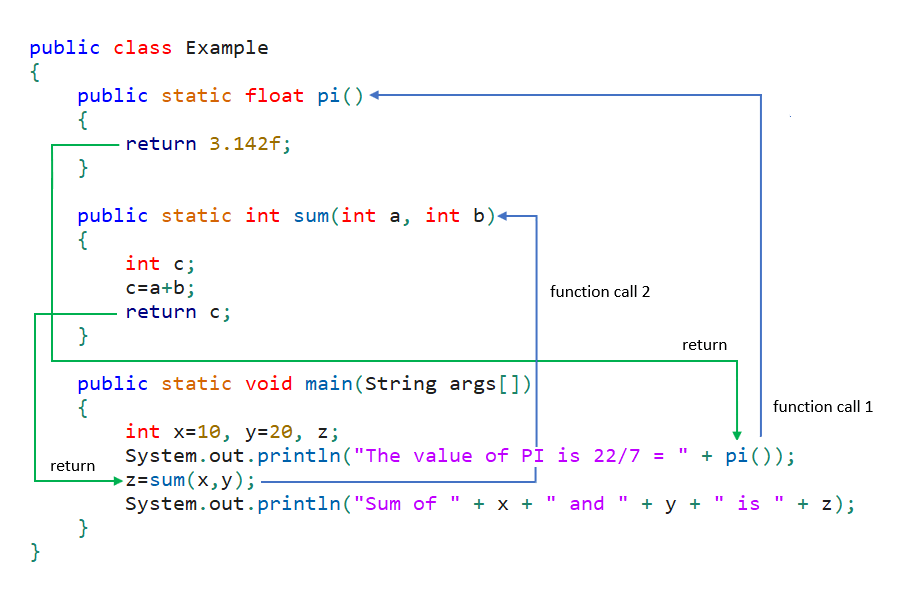
In the above diagram, we see that when the main program calls the function pi(), the program flow goes to the body of the function pi() and execute its codes. After that, it returns the value to the main program and then calls the second function sum() by sending it two arguments (x,y), the program flow goes to the body of the function sum(), and execute its code and then returns the value to the main program. At last, the main programs end.
Passing Different Types of Arguments in Function
We can pass different types of arguments in a function like an integer, float, array, etc. Let's see some examples.
Example 1 (passing integers and float)
import java.util.Scanner;
public class Example
{
public static void simpleinterest(int p,float r,int t)
{
float si;
si=(p*r*t)/100;
System.out.println("Simple Interest = " + si);
}
public static void main(String args[])
{
int principal,time;
float rate;
Scanner sc=new Scanner(System.in);
System.out.print("Enter principal amount: ");
principal=sc.nextInt();
System.out.print("Enter yearly rate %: ");
rate=sc.nextFloat();
System.out.print("Enter time in year: ");
time=sc.nextInt();
simpleinterest(principal,rate,time);
}
}
Output
Enter principal amount: 5000 Enter yearly rate : 6.25 Enter time in year: 1 Simple Interest = 312.5
Example 2 (passing one dimensional integer array)
import java.util.Scanner;
public class Example
{
public static void sum(int arr[])
{
int s=0,i;
for(i=0; i<5; i++)
{
s=s+arr[i];
}
System.out.println("Sum of Numbers = " + s);
}
public static void main(String args[])
{
int n[]=new int[5], i;
Scanner sc=new Scanner(System.in);
System.out.println("Enter 5 numbers");
for(i=0; i<5; i++)
{
n[i]=sc.nextInt();
}
sum(n);
}
}
Output
Enter 5 numbers 5 8 2 4 6 Sum of Numbers = 25
In the above program, the variable int arr[] represents a one dimensional integer array argument in the function sum(). We can use any other variable name in place of arr.
Example 3 (passing two dimensional integer array)
import java.util.Scanner;
public class Example
{
public static void sum(int num[][])
{
int s=0,i,j;
for(i=0; i<3; i++)
{
for(j=0; j<3; j++)
{
s=s+num[i][j];
}
}
System.out.println("Sum of Numbers = " + s);
}
public static void main(String args[])
{
int n[][]=new int[3][3], i,j;
Scanner sc=new Scanner(System.in);
System.out.println("Enter 9 numbers");
for(i=0; i<3; i++)
{
for(j=0; j<3; j++)
{
n[i][j]=sc.nextInt();
}
}
sum(n);
}
}
Output
Enter 9 numbers 12 6 8 9 14 18 21 11 5 Sum of Numbers = 104
In the above program, the variable int num[][] represents a two dimensional integer array argument in the function sum().
Formal and Actual Arguments
The arguments or parameters used during function declaration within the round brackets ( ) are known as Formal Arguments. Whereas the arguments or parameters that are used when providing input to the function from the main program are known as Actual Arguments. Let's see an example for more understanding.
Example
import java.util.Scanner;
public class Example
{
public static float simpleinterest(int p,float r,int t)
{
float si;
si=(p*r*t)/100;
return si;
}
public static void main(String args[])
{
int principal,time;
float rate,interest;
Scanner sc=new Scanner(System.in);
System.out.print("Enter principal amount: ");
principal=sc.nextInt();
System.out.print("Enter yearly rate %: ");
rate=sc.nextFloat();
System.out.print("Enter time in year: ");
time=sc.nextInt();
interest=simpleinterest(principal,rate,time);
System.out.println("Simple Interest = " + interest);
}
}
In the above program, variable p, r and t in function simpleinterest() are used to receive inputs from the main program, so these variables are known as Formal Argument.
The variables principal, rate and time used in the main program to provide inputs to the function simpleinterest() are known as Actual Arguments because they provide the actual inputs to the function simpleinterest().
Pass by Value or Call by Value
In Pass by Value the values of the variables are passed to the formal arguments of the function. In this case the values of the actual arguments are not affected by changing the values of the formal arguments. See the example given below.
Example of Pass by Value
import java.util.Scanner;
public class Example
{
public static void changevalue(int a,int b)
{
a=a+2;
b=b+2;
System.out.println("In function changes are " + a + " and " + b);
}
public static void main(String args[])
{
int x=10,y=20;
System.out.println("Before calling the function");
System.out.println("x=" + x + " and y=" + y);
changevalue(x,y);
System.out.println("After calling the function");
System.out.println("x=" + x + " and y=" + y);
}
}
Output
Before calling the function x=10 and y=20 In function changes are 12 and 22 After calling the function x=10 and y=20
In the above program, the variable x and y are passed as arguments to the function changevalue(). The value of x and y is passed to a and b. The memory location of x and y are different from the memory location of a and b. Hence, when the value of a and b is incremented, there will be no effect on the value of x and y. So after calling the function the value of x and y are the same as before calling the function.
Note: Java support only pass by value or call by value. Unlike C and C++, java does not support pass by reference or call by reference.
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
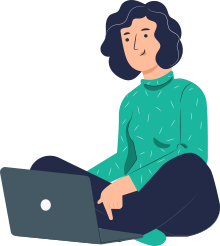