Naming Convention in Java Programming Language
Java Basic Concepts
In this lesson, we will learn about the Naming Convention in the Java programming language. We will go through the rules of naming Variables, Class, Interface, Methods, and Package. Along with some examples and a quiz on it.
What is Naming Conventions
In Java programming, naming conventions are set of rules for choosing the valid name to be used for variable, class, package, interface and function in a java program.
Naming Conventions rules for Variables are:
- It should begin with an alphabet.
- There may be more than one alphabet, but without any spaces between them.
- Digits may be used but only after alphabet.
- No special symbol can be used except the underscore (_) and currency ($) symbol. When multiple words are needed, an underscore should separate them.
- No keywords or command can be used as a variable name.
- All statements in java language are case sensitive. Thus a variable A (in uppercase) is considered different from a variable declared a (in lowercase).
Now let's see some examples for more understanding.
Example 1
It should begin with an alphabet.
x // x is a valid variable name because it starts with an alphabet x
Example 2
There may be more than one alphabet, but without any spaces between them.
total // total is a valid variable name as there is no space between alphabets
Example 3
Digits may be used but only after alphabet.
ar15 // ar15 is a valid variable name as digits have been used after the alphabet
a6b2 // a6b2 is a valid variable name as digits have been used after the alphabet
Example 4
No special symbol should be present within the variable name except underscore _.
total_cost // total_cost is a valid variable name as there is an underscore
total cost // total cost is an invalid variable name as there is a space
total-cost // total-cost is an invalid variable name as there is a hyphen
total$ // total$ is a valid variable name as there is a dollar symbol
Example 5
No keywords or command can be used as a variable name.
for // here for is an invalid variable name because it is a keyword in Java
if // if is an invalid variable name because it is a keyword in Java
case // case is an invalid variable name because it is a keyword in Java
final // final is an invalid variable name because it is a keyword in Java
Example 6
All statements in Java language are case sensitive. Thus a variable A (in uppercase) is considered different from a variable declared a (in lowercase).
a // a is a valid variable written in lowercase
A // A is a valid variable written in uppercase so both are different variables
Naming Conventions rules for Class and Interface are:
- It should begin with an uppercase letter.
- There may be more than one alphabet, but without any spaces between them.
- No special symbol can be used.
- No keywords or command can be used as a class or interface name.
- If the class name is more than one word then the first letter of each word should be written in uppercase and the rest of the letter should be written in lowercase.
Example
Scanner // it is a 1 word class name
BufferedReader // it is a 2 words class name
InputStreamReader // it is a 3 words class name
ServerNotActiveException // it is a 4 words class name
Naming Conventions rules for Methods (Functions) are:
- It should begin with a lowercase letter.
- There may be more than one alphabet, but without any spaces between them.
- No special symbol can be used.
- No keywords or command can be used as a method name.
- If the method name is more than one word then the first word should be written in lowercase and remaining words' first letter should be written in uppercase and the rest of letters should be written in lowercase.
Example
length // it is a 1 word name
readLine // it is a 2 words name
toUpperCase // it is a 3 words name
compareToIgnoreCase // it is a 4 words name
Naming Conventions rules for Package are:
- It should written in a lowercase letter.
- There may be more than one alphabet, but without any spaces between them.
- No special symbol can be used.
- No keywords or command can be used as a package name.
- If the package name is more than one word then all the words should be written in lowercase without any space between them.
Example
util // it's name of a package in Java
applet // it's name of a package in Java
beans // it's name of a package in Java
security // it's name of a package in Java
gamearea // it's a 2 words valid package name
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
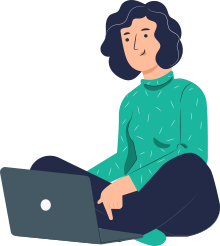