Two Dimensional Array in Java Programming
Arrays in Java
In this lesson, we will understand what is Two Dimensional Array in Java Programming along with some examples.
What is Two Dimensional Array (2D Array) in Java
A Two Dimensional Array in Java is a collection of 1D Array. It consists of rows and columns and looks like a table. A 2D array is also known as Matrix.
Declaration Syntax of a Two Dimensional Array in Java
datatype variable_name[][] = new datatype[row_size][column_size];
Or
datatype[][] variable_name = new datatype[row_size][column_size];
Here row_size is the number of rows we want to create in a 2D array and, column_size is the number of columns in each row.
Example
int a[][]=new int[3][3];
Or
int[][] a=new int[3][3];
Once we declare the 2D Array, it will look like as shown in the picture below:
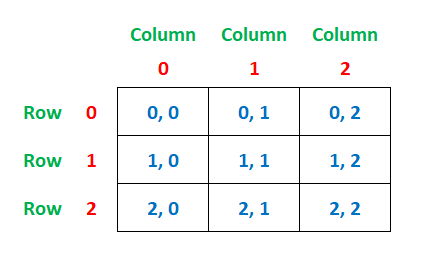
In the above image, you can see that we have created a 2D Array having 3 rows and 3 columns. We can call the above array as a 3x3 Matrix.
The first row and column always start with index 0.
Note: A 2D array is used to store data in the form of a table.
Declaration and Initialization of a Two Dimensional Array in Java
In Java programming a two dimensional array can be declared and initialized in several ways. Let’s see the different ways of initializing a 2D array.
Example 1:
int a[][]= new int[][] {{1,2,3}, {4,5,6}, {7,8,9}};
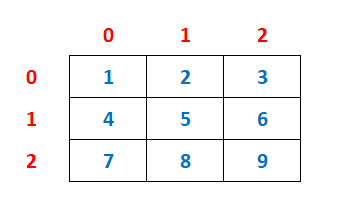
Example 2:
int a[][]= {{15,27,36}, {41,52,64}, {79,87,93}};
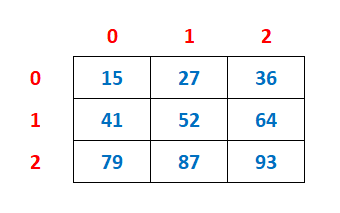
Example 3: (All cell contains the value 0)
int a[][]= new int[3][3];
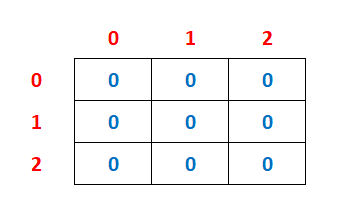
Store Numbers in a Two Dimensional Array
To store the number in each cell of the 2D array we can use the following syntax.
array_name[row_index][column_index]=value;
Example
a[0][0]=15;
a[0][1]=27;
a[0][2]=36;
a[1][0]=41;
a[1][1]=52;
a[1][2]=64;
a[2][0]=79;
a[2][1]=87;
a[2][2]=93;
Access Numbers in a Two Dimensional Array
We can access any number stored in a 2D array using the following syntax.
array_name[row_index][column_index]
Example
System.out.println(a[0][0]+" "+a[0][1]+" "+a[0][2]);
System.out.println(a[1][0]+" "+a[1][1]+" "+a[1][2]);
System.out.println(a[2][0]+" "+a[2][1]+" "+a[2][2]);
Output
15 27 36 41 52 64 79 87 93
Store and Access the Numbers in a 2D Array using Loops
We can also store as well as access the numbers in a 2D array using either for, while or do while loop. Let's see a few examples.
Example 1
Program to input numbers in a 3x3 Matrix and display the numbers in a table format.
import java.util.Scanner;
public class Example
{
public static void main(String args[])
{
int a[][]=new int[3][3];
Scanner sc=new Scanner(System.in);
int r,c;
System.out.println("Enter 9 numbers");
for(r=0; r<3; r++)
{
for(c=0; c<3; c++)
{
a[r][c]=sc.nextInt();
}
}
System.out.println("\nOutput");
for(r=0; r<3; r++) // this loop is for row
{
for(c=0; c<3; c++) // this loop will print 3 numbers in each row
{
System.out.print(a[r][c]+" ");
}
System.out.println(); // break the line after printing the numbers in a row
}
}
}
Output
Enter 9 numbers 84 36 41 98 34 57 20 31 24 Output 84 36 41 98 34 57 20 31 24
Here, you can see that we have run a nested for loop to store the user's input in a 3x3 matrix. After that we have run another nested for loop to access each number from the 2D array and print on the screen in a table format.
Example 2
Program to input numbers in a 3x3 Matrix and print only even numbers if present in the matrix.
import java.util.Scanner;
public class Example
{
public static void main(String args[])
{
int a[][]=new int[3][3];
Scanner sc=new Scanner(System.in);
int r,c;
System.out.println("Enter 9 numbers");
for(r=0; r<3; r++)
{
for(c=0; c<3; c++)
{
a[r][c]=sc.nextInt();
}
}
System.out.println("\nEven Numbers");
for(r=0; r<3; r++)
{
for(c=0; c<3; c++)
{
if(a[r][c]%2==0)
{
System.out.print(a[r][c]+" ");
}
}
}
}
}
Output
Enter 9 numbers 84 36 41 98 34 57 20 31 24 Even Numbers 84 36 98 34 20 24
Here, you can see that we have entered numbers in a 3x3 matrix using nested for loop. After that we have run another nested for loop to fetch the numbers from the 2D array and print only those numbers which are divisible by 2.
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
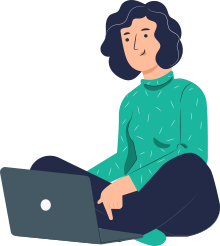