Arithmetic Operators in Python Programming
Operators in Python
In this lesson, we are going to look at what is arithmetic operators and will learn the different types of arithmetic operator with the help of examples.
What is Arithmetic Operators
Arithmetic Operators in Python are used to performing arithmetic or mathematical operations on operands.
There are 7 types of arithmetic operators:
- + Addition
- - Subtraction
- *Multiplication
- / Division
- % Modulus
- ** Exponentiation
- // Floor Division
+ Addition Operator
+ Addition Operator is used for adding the value of two or more variables. Let's see the example for more understanding.
Example
x=5
y=2
z=x+y # value of z becomes 7
In the example above the value of x and y is first added and then stored in z. So the result of z is 7.
- Subtraction Operator
- Subtraction Operator is used for subtracting the value of two or more variables. Let's see the example for more understanding.
Example
x=5
y=2
z=x-y # value of z becomes 3
In the example above the value of y is subtracted from x and the final result is stored in z. So the result of z is 3.
* Multiplication Operator
* Multiplication Operator is used for multiplying the value of two or more variables. Let's see the example for more understanding.
Example
x=5
y=2
z=x*y # value of z becomes 10
In the example above the value of x and y is first multiplied and then stored in z. So the result of z is 10.
/ Division Operator
/ Division Operator is used for dividing the value of two or more variables and the quotient is returned as the result of the division. Let's see the example for more understanding.
Example
x=5
y=2
z=x/y # value of z becomes 2.5 which is a quotient
In the example above the value of x and y is first divided and then stored the quotient in z. So the result of z is 2.5.
% Modulus Operator
% Modulus Operator is used for dividing the value of two or more variables and the remainder is returned as the result of the division. Let's see the example for more understanding.
Example
x=5
y=2
z=x%y # value of z becomes 1 which is a remainder
In the example above the value of x and y is first divided and then stored the remainder in z. So the result of z is 1.
** Exponentiation Operator
** Exponentiation Operator is used to multiply a number till a certain number of times. In mathematics it is written as xn where x is the base number and n is the power, So x will be multiplied n times. Let's see the example for more understanding.
Example
x=5
y=2
z=x**y # value of z becomes 25
In the example above the value of x is multiplied two times (value of y) and then stored the result in z. So the result of z is 25.
// Floor Division Operator
// Floor Division Operator is used to round off the result of the division down to the nearest whole number. In other words, the decimal portion is removed from the result after division. Let's see the example for more understanding.
Example
x=27
y=4
z=x//y # value of z becomes 6
In the example above the value of x and y is first divided and then the decimal portion is removed from the quotient and after that the value is stored in z. So the result of z is 6.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
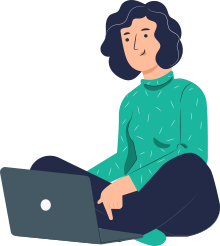