String Methods in Python Programming
String in Python
In this lesson, we will learn about the different types of string methods in Python programming and their uses with the help of examples.
What are String Methods in Python
String methods in Python are a collection of useful functions applied on a string to solve various string related questions. Let's see all the important string methods one by one with examples.
find() Method
The find() method returns the index of the first occurrence of the specified character or string within the current string. The find() method accepts two arguments.
The first argument is the string or character and the second optional argument is the index from where we want to check the occurrence. If the index is not given, it will start checking the occurrence from index 0.
If search string or character is not found in the current string then the find() method will return -1.
Example
str='I am a boy and I love java programming'
if str.find('I')>=0:
print("Found at postion", str.find('I'))
else:
print("Not found")
if str.find('I',5)>=0:
print("Found at postion", str.find('I',5))
else:
print("Not found")
Output
Found at postion 0 Found at postion 15
rfind() Method
The rfind() method returns the index of the last occurrence of the specified character or string within the current string.
Example
str='I am a boy and I love java programming'
if str.rfind('a')>=0:
print("Last found at index", str.rfind('a'))
else:
print("Not found")
Output
Last found at index 32
count() Method
The count() method returns the number of times a specified character or string occurs within the current string.
The count() method accepts three arguments. The first argument is the character or string we are counting for, and the optional second and third arguments are the start and end range to count within.
Example
str='hello world, hello python, hello programming, hello coding'
print(str.count('hello'))
print(str.count('hello',12))
print(str.count('hello',12,45))
Output
4 3 2
startswith() Method
The startswith() method returns True if the current string starts with the specified string, otherwise, it returns False.
The startswith() method accepts three arguments. The first argument is the character or string we are checking for, and the optional second and third arguments are the start and end range to check within.
Example
str='hello world, hello python, hello programming, hello coding'
print(str.startswith('hello'))
print(str.startswith('hello',13))
print(str.startswith('hello',19,45))
Output
True True False
endswith() Method
The endswith() method returns True if the current string ends with the specified string, otherwise, it returns False.
The endswith() method accepts three arguments. The first argument is the character or string we are checking for, and the optional second and third arguments are the start and end range to check within.
Example
str='hello world, hello python, hello programming, hello coding'
print(str.endswith('coding'))
print(str.endswith('programming',24))
print(str.endswith('python',6,25))
Output
True False True
isalnum() Method
The isalnum() method returns True if the string is an alphanumeric (contains only alphabets and numbers), otherwise, it returns False. The string should not contain any space or other special character.
Example
str1='section2b'
str2='section 2b'
print(str1.isalnum())
print(str2.isalnum())
Output
True False
isalpha() Method
The isalpha() method returns True if all characters in the string are alphabets, otherwise, it returns False.
Example
str1='sectionb'
str2='section-b'
print(str1.isalpha())
print(str2.isalpha())
Output
True False
isdigit() Method
The isdigit() method returns True if all characters in the string are digits, otherwise, it returns False.
Example
str1='2586'
str2='2586a'
print(str1.isdigit())
print(str2.isdigit())
Output
True False
islower() Method
The islower() method returns True if all alphabets in the string are lowercase letter, otherwise, it returns False.
Example
str1='apple ball'
str2='2-apple 1-ball'
print(str1.islower())
print(str2.islower())
Output
True True
isupper() Method
The isupper() method returns True if all alphabets in the string are uppercase letter, otherwise, it returns False.
Example
str1='APPLE BALL'
str2='2-APPLE 1-BALL'
print(str1.isupper())
print(str2.isupper())
Output
True True
lower() Method
The lower() method returns a new string after converting all the characters of the string in lowercase.
Example
str='PYTHON'
print(str.lower())
Output
python
upper() Method
The upper() method returns a new string after converting all the characters of the string in uppercase.
Example
str='python'
print(str.upper())
Output
PYTHON
replace() Method
The replace() method returns a new string after replacing all the occurrences of a specified character or string with the other specified character or string. The replace() methods accepts three arguments.
The first argument is the old value, the second argument is the new value and the optional third argument is the number of times to replace the old value with the new value.
Example
str='My dog name is max. My dog loves to eat meat every day.'
print(str.replace('a','p'))
print(str.replace('dog','cat'))
print(str.replace('a','p',2))
Output
My dog npme is mpx. My dog loves to ept mept every dpy. My cat name is max. My cat loves to eat meat every day. My dog npme is mpx. My dog loves to eat meat every day.
strip() Method
The strip() method returns a new string after removing all the whitespaces from beginning and end of the string. The strip() methods accepts one optional argument.
The optional argument is a set of characters to remove as leading or trailing characters.
Example
str1=' Hello World '
str2='********Hello World********'
print(str1.strip())
print(str2.strip('*'))
Output
Hello World Hello World
split() Method
The strip() method returns a list after splitting the string at the specified separator. The default separator is any whitespace character.
Example
str1='Hello how are you'
str2='apple-banana-orange-cherries'
print(str1.split())
print(str2.split('-'))
Output
['Hello', 'how', 'are', 'you'] ['apple', 'banana', 'orange', 'cherries']
capitalize() Method
The capitalize() method returns a new string after converting the first letter of the string in uppercase.
Example
str1='hello how are you'
str2='14 years old boy'
print(str1.capitalize())
print(str2.capitalize())
Output
Hello how are you 14 years old boy
title() Method
The title() method returns a new string after converting the first letter of each word of the string in uppercase.
Example
str1='hello how are you'
str2='14 years old boy'
print(str1.title())
print(str2.title())
Output
Hello How Are You 14 Years Old Boy
swapcase() Method
The swapcase() method returns a new string after converting the uppercase letter into lowercase and vice versa.
Example
str='heLLo HoW ARe yOu'
print(str.swapcase())
Output
HEllO hOw arE YoU
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
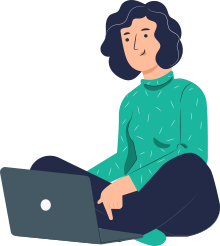