Arrays in Python Programming
Data Structures in Python
In this lesson, we will understand what is Array in Python Programming along with some examples.
What is Array in Python?
An Array in Python programming is a special type of variable that can store multiple values of only a single data type such as integer, floating point numbers, character, etc. at a contagious location in computer memory. Here contagious location means at a fixed gap in computer memory.
Arrays can increase or decrease their size dynamically whenever elements are added or removed from the array.
Arrays are mutable. It means that we can modify the elements of an Array directly.
Suppose we want to store the age of 10 students. In that case, we have to declare 10 variables in our Python program to store the age of 10 students.
Now here comes the use of a an array. With the help of an array, we will declare a single variable in our Python program that can store the age of 10 students at a time.
Declaration Syntax of an Array in Python
array_name = array('typecode', [elements])
Here, typecode specifies which type of data we want to store in the array.
Example
a = array('i', [5,8,6,2,9])
Here typecode 'i' represents an integer type array where we can store only integer numbers. Below table summarizes the different type codes used for defining arrays in python.
Type Codes to Create Arrays
Typecode | C++ Type | Python Type | Minimum Size in Bytes |
'b' | signed char | int | 1 |
'B' | unsigned char | int | 1 |
'u' | Py_UNICODE | unicode character | 2 |
'h' | signed short | int | 2 |
'H' | unsigned short | int | 2 |
'i' | signed int | int | 2 |
'I' (Capital i) | unsigned int | long | 2 |
'l' (small L) | signed long | int | 4 |
'L' | unsigned long | long | 4 |
'f' | float | float | 4 |
'd' | double | float | 8 |
Importing the Array Module
Before creating an array, we have to import the array module in our python program, as shown below.
Example
from array import *
a=array('i', [5,8,6,2,9])
Creating an Integer Type Array
A python program to show how to create and display an integer type array in python.
Example
from array import *
a=array('i', [15,2,49,51,86])
# printing the elements from an integer type array
for x in a:
print(x)
Output
15 2 49 51 86
Creating a Double Type Array
A python program to show how to create and display a double type array in python.
Example
from array import *
a=array('d', [18.23,24.11,564.2369,44,98.513])
# printing the elements from a double type array
for x in a:
print(x)
Output
18.23 24.11 564.2369 44.0 98.513
Creating a Character Type Array
A python program to show how to create and display a character type array in python.
Example
from array import *
a=array('u', ['a','e','i','o','u'])
# printing the elements from a character type array
for x in a:
print(x)
Output
a e i o u
Create an Array from another Array
A python program to show how to create one array from another array.
Example
from array import *
ar1=array('i', [15,2,49,51,86])
ar2=array(ar1.typecode, ar1)
for n in ar2:
print(n)
Output
15 2 49 51 86
Creating an Empty Array
We can also create an empty array that does not contain elements at the time of declaration. See the example given below.
Example
from array import *
a=array('i',[]) # An empty array
print(a)
Output
array('i')
Array Slicing
Array slicing is the process of accessing a part of the array using either positive or negative indexing with the help of array slicing syntax given below.
array_name[ start : end+1 : step]
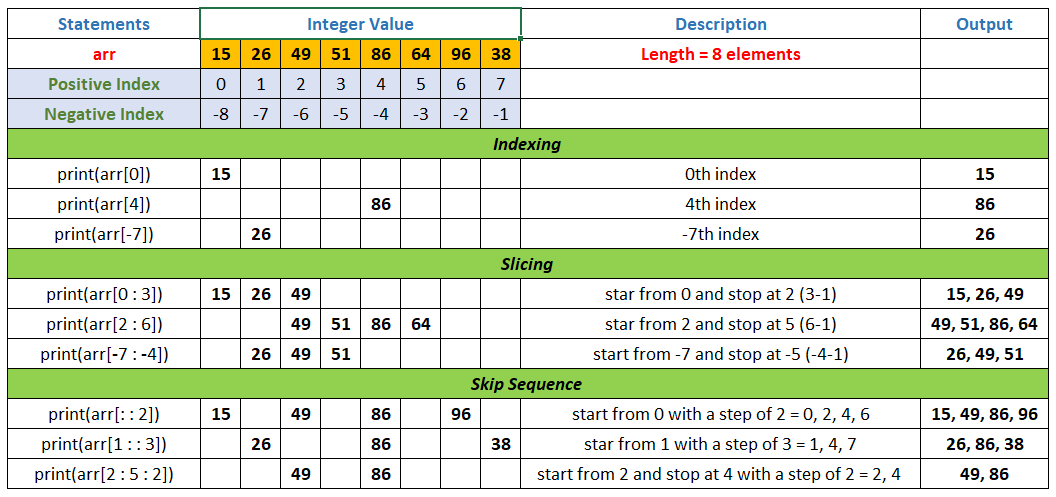
Example
from array import *
arr=array('i', [15,26,49,51,86,64,96,38])
print(arr[0])
print(arr[4])
print(arr[-7])
print(arr[0:3])
print(arr[2:6])
print(arr[-7:-4])
print(arr[::2])
print(arr[1::3])
print(arr[2:5:2])
Output
15 86 26 array('i', [15, 26, 49]) array('i', [49, 51, 86, 64]) array('i', [26, 49, 51]) array('i', [15, 49, 86, 96]) array('i', [26, 86, 38]) array('i', [49, 86])
Modifying Elements of an Array
We can modify an element of an array just by assigning a new value to the element's index as shown below.
Example
from array import *
a=array('i',[15,2,49,51,86])
a[0]=12
a[2]=37
# printing the array after modifying the elements at index 0 and 2
print(a)
Output
array('i', [12, 2, 37, 51, 86])
Iterate all Elements in an Array using range() Function
We can also iterate all elements in an array using range() function as shown below.
Example
from array import *
a=array('i', [15,2,49,51,86])
# printing the elements from an integer type array
for i in range(0,len(a)):
print(a[i])
Output
15 2 49 51 86
Note: Here len() method is used to get the total number of elements present in the array. The index of the first element in the array starts from 0.
Array Methods
Array methods in Python are a collection of useful functions used to perform a specific task on an array. Let's see all the important array methods one by one with examples.
append() Method
The append() method adds an element at the end of an existing array.
Example
from array import *
a=array('i', [11,9,26,34,53])
print('Original Array: ',a)
a.append(88)
print('Array after appending 88: ',a)
Output
Original Array: array('i', [11, 9, 26, 34, 53]) Array after appending 88: array('i', [11, 9, 26, 34, 53, 88])
extend() Method
The extend() method appends another array at the end of an existing array.
Example
from array import *
a=array('i', [15,2,49,51,86])
b=array('i', [9,7,5,62])
print('Array a: ',a)
print('Array b: ',b)
a.extend(b)
print('Array a after appending array b: ',a)
Output
Array a: array('i', [15, 2, 49, 51, 86]) Array b: array('i', [9, 7, 5, 62]) Array a after appending array b: array('i', [15, 2, 49, 51, 86, 9, 7, 5, 62])
count() Method
The count() method returns the number of occurrences of a specified element in an existing array.
Example
from array import *
a=array('i', [11,9,26,34,26,84,97,26])
print('Original Array: ',a)
print('Total occurrence of element 26: ',a.count(26))
Output
Original Array: array('i', [11, 9, 26, 34, 26, 84, 97, 26]) Total occurrence of element 26: 3
index() Method
The index() method returns the index of the first occurrence of the specified element in an existing array. The index() method raise ValueError if the element is not found.
Example
from array import *
a=array('i', [11,9,26,34,26,84,97,26])
print('Original Array: ',a)
print('Index of first occurrence of element 26: ',a.index(26))
Output
Original Array: array('i', [11, 9, 26, 34, 26, 84, 97, 26]) Index of first occurrence of element 26: 2
insert() Method
The insert() method inserts an element at a specific index in an existing array.
Example
from array import *
a=array('i', [11,9,26,34,84])
print('Original Array: ',a)
a.insert(2, 59)
print('Array after inserting 59 at index 2: ',a)
Output
Original Array: array('i', [11, 9, 26, 34, 84]) Array after inserting 59 at index 2: array('i', [11, 9, 59, 26, 34, 84])
pop() Method
The pop() method removes and returns the last element of an array if the element's index is not specified. To remove a specific element from the array, we have to specify its index.
Example
from array import *
a=array('i', [11,9,26,34,84])
print('Original Array: ',a)
a.pop()
print('Array after removing the last element: ',a)
a.pop(2)
print('Array after removing the element at index 2: ',a)
Output
Original Array: array('i', [11, 9, 26, 34, 84]) Array after removing the last element: array('i', [11, 9, 26, 34]) Array after removing the element at index 2: array('i', [11, 9, 34])
remove() Method
The remove() method removes the first occurrence of the specified element in an existing array. The remove() method raise ValueError if the element is not found.
Example
from array import *
a=array('i', [11,9,26,34,26,84,97,26])
print('Original Array: ',a)
a.remove(26)
print('Array after removing the first occurrence of element 26: ',a)
Output
Original Array: array('i', [11, 9, 26, 34, 26, 84, 97, 26]) Array after removing the first occurrence of element 26: array('i', [11, 9, 34, 26, 84, 97, 26])
reverse() Method
The reverse() method reverses the order of element in an existing array.
Example
from array import *
a=array('i', [11,9,26,34,84,97])
print('Original Array: ',a)
a.reverse()
print('Array after reversing the order of elements: ',a)
Output
Original Array: array('i', [11, 9, 26, 34, 84, 97]) Array after reversing the order of elements: array('i', [97, 84, 34, 26, 9, 11])
Create an Integer Array from User Input
A python program to show how to create an integer array from user input.
Example
from array import *
a=array('i',[])
print('Enter 5 numbers')
for i in range(5):
a.append(int(input()))
print(a)
Output
Enter 5 numbers 12 8 47 6 91 array('i', [12, 8, 47, 6, 91])
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
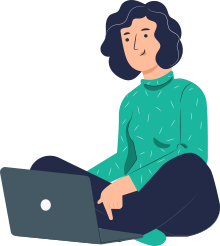