Relational or Comparison Operators in Python
Operators in Python
In this lesson, we will learn what is the relational or comparison operator and how it works in Python programming with some examples.
What is Relational Operators
Relational Operators which is also known as Comparision Operators are used for comparing the values of two operands (means variable or value).
For example: checking if one operand is equal to the other operand or not or if one operand is greater than the other operand or not etc.
There are 6 types of relational operators in Python and they are:
- == Equal to Equal to
- > Greater than
- < Less than
- >= Greater than or equal to
- <= Less than or equal to
- != Not equal to
Now let's see the examples of all the relational operators one by one for more understanding.
== Equal to equal to
== Equal to equal to relational operator is used for checking if the value of two operands is the same or not. The output of == relational operator is True if the relation is correct otherwise the output will be False if the relationship is wrong.
Example
x=5
y=5
z=6
a=x==y # output of a is True
b=x==z # output of b is False
In the example above the output of a is True because the value of x and y is the same so, the relation is correct. On the other hand, the output of b is False because the value of x and z is not the same so the relationship is wrong.
> Greater than
> Greater than relational operator is used for checking if the value of the left operand is greater then the value of the right operand. The output of > relational operator is True if the relation is correct otherwise the output will be False if the relationship is wrong.
Example
x=8
y=5
z=10
a=x>y # output of a is True
b=x>z # output of b is False
In the example above the output of a is True because the value of x is greater than the value of y so, the relation is correct. On the other hand, the output of b is False because the value of x is not greater than the value of z so the relationship is wrong.
< Less than
< Less than relational operator is used for checking if the value of the left operand is less then the value of the right operand. The output of < relational operator is True if the relation is correct otherwise the output will be False if the relationship is wrong.
Example
x=5
y=8
z=3
a=x<y # output of a is True
b=x<z # output of b is False
In the example above the output of a is True because the value of x is less than the value of y so, the relation is correct. On the other hand, the output of b is False because the value of x is not less than the value of z so the relationship is wrong.
>= Greater than or equal to
>= Greater than or equal to relational operator is used for checking if the value of the left operand is greater then or equal to the value of the right operand. The output of >= relational operator is True if the relation is correct otherwise the output will be False if the relationship is wrong.
Example
w=5
x=5
y=3
z=10
a=w>=x # output of a is True
b=x>=y # output of b is True
c=y>=z # output of c is False
In the example above the output of a is True because the value of w is not greater but equal to the value of x so, the relation is correct. Next, the output of b is True because the value of x is greater than the value of y so, the relationship is correct. On the other hand, the output of c is False because the value of y is neither greater nor equal to the value of z so the relationship is wrong.
<= Less than or equal to
<= Less than or equal to relational operator is used for checking if the value of the left operand is less then or equal to the value of the right operand. The output of <= relational operator is True if the relation is correct otherwise the output will be False if the relationship is wrong.
Example
w=5
x=5
y=7
z=3
a=w<=x # output of a is True
b=x<=y # output of b is True
c=y<=z # output of c is False
In the example above the output of a is True because the value of w is not less but equal to the value of x so, the relation is correct. Next, the output of b is True because the value of x is less than the value of y so, the relationship is correct. On the other hand, the output of c is False because the value of y is neither less nor equal to the value of z so the relationship is wrong.
!= Not equal to
!= Not equal to relational operator is used for checking if the value of the left operand is not equal to the value of the right operand. The output of != relational operator is True if the relation is correct otherwise the output will be False if the relationship is wrong.
Example
x=5
y=8
z=5
a=x!=y # output of a is True
b=x!=z # output of b is False
In the example above the output of a is True because the value of x is not equal to the value of y so, the relation is correct. On the other hand, the output of b is False because the value of x is equal to the value of z so the relationship is wrong.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
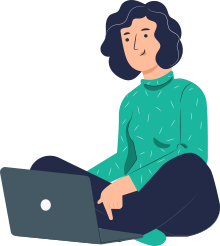