Identity Operators in Python
Operators in Python
In this lesson, we will learn what is the Identity Operators and how it works in Python programming with some examples.
What is Identity Operators
Identity Operators are used in Python to check if two values (or variables) are located on the same part of the memory or not. Two variables whose values are equal does not mean that they are same.
There are 2 types of identity operators in Python and they are:
- is
- is not
Now let's see the examples of the identity operators one by one for more understanding.
is Operator
is operator is used to check if the two variables are identical (refer to the same object). If they are identical then the result will be True otherwise the result will be False.
Example
a=15
b=15
c="apple"
d="apple"
e=[1,2,3]
f=[1,2,3]
x=a is b # output of x is True
y=c is d # output of y is True
z=e is f # output of z is False
In the example above the output of x is True because both the variables a and b are integer type and their values are also the same. Similarly, the output of y is True because both the variables c and d are string type and their values are also the same. Whereas the output of z is False because both the variables e and f are list and the interpreter stored them in a different memory location.
So being their values are the same but as they are not stored, in the same memory location so, they are not identical.
is not Operator
is not operator is used to check if the two variables are not identical (do not refer to the same object). If they are not identical then the result will be True otherwise the result will be False.
Example
a=15
b=15
c="apple"
d="ball"
e=[1,2,3]
f=[1,2,3]
x=a is not b # output of x is False
y=c is not d # output of y is True
z=e is not f # output of z is True
In the example above the output of x is False because both the variables a and b are integer type and their values are also the same. Whereas, the output of y is True because both the variables c and d are string type but their values are not the same. Similarly, output of z is True because both the variables e and f are list and the interpreter stored them in a different memory location.
So being their values are the same but as they are not stored, in the same memory location so, they are not identical.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
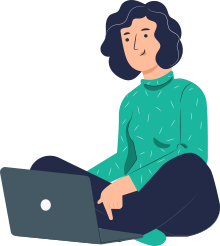