Dictionaries in Python Programming
Data Structures in Python
In this lesson, we will understand what is Dictionaries in Python Programming along with some examples.
What is Dictionaries in Python?
A Dictionary is an ordered collection of some key:value pairs. The key and its value is separated by a colon (:).
All the key:value pairs are inserted within a curly braces { } separated by commas (,).
Dictionaries are mutable we can add or remove key:value pair in a dictionary once it is created.
Note: Dictionaries are ordered from Python version 3.7 onwards and unordered below version 3.7.
Declaration Syntax of a Dictionary in Python
dictionary_name = {key:value, key:value,....}
Example
# A dictionary containing an employee details
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
Adding Elements to a Dictionary
We can add elements (key:value pair) to a dictionary by assignment as per the following syntax.
Syntax of Adding an Element to a Dictionary
variable_name[key] = value
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
emp['city']='California'
print(emp)
Output
{'id': 'e156', 'name': 'Allen', 'age': 34, 'salary': 15000, 'city': 'california'}
Updating Element of a Dictionary
We can update the value of an existing key in a dictionary by assigning a new value to the same key.
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
emp['age']=42
print(emp)
Output
{'id': 'e156', 'name': 'Allen', 'age': 42, 'salary': 15000}
In the above example, we can see that the value of the key age has changed from 34 to 42 because we have used assigned a new value to the key.
Accessing an Element of a Dictionary
In a dictionary, an element or value is accessed by its key using the syntax given below.
Syntax of Accessing an Element of a Dictionary
variable_name = dictionary_name[key]
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
x=emp['name']
print(x)
Output
Allen
Deleting an Element from a Dictionary
We can delete an element (key:value pair) from a dictionary by using del statement as follows.
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
del emp['salary']
print(x)
Output
{'id': 'e156', 'name': 'Allen', 'age': 34}
Traversing All Elements of a Dictionary
We can traverse or access all the elements of a dictionary one by one with the help of for loop as shown below.
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
for key in emp:
print(key,':',emp[key])
Output
id : e156 name : Allen age : 34 salary : 15000
Creating an Empty Dictionary
We can also create an empty dictionary that does not contain elements at the time of declaration. See the example given below.
Example
a = {} # An empty dictionary
b = dict() # Another way of creating an empty dictionary
print(a)
print(b)
Output
{} {}
Use of Membership Operator in Dictionary
Both in and not in operators works on Dictionary just like they work for other sequences such as strings, lists, tuples and sets. The in operator tells if a key is present in the dictionary or not and not in does the opposite. See the example given below.
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
print(emp)
if ('name' in emp) == True:
print('name key is prenest in the dictionary')
else:
print('name key is not prenest in the dictionary')
if ('city' not in emp) == True:
print('city key is not prenest in the dictionary')
else:
print('city key is prenest in the dictionary')
Output
{'id': 'e156', 'name': 'Allen', 'age': 34, 'salary': 15000} name key is prenest in the dictionary city key is not prenest in the dictionary
Dictionary Methods and Functions
Dictionary methods in Python are a collection of useful functions used to perform a specific task on a dictionary.
The difference between Function and Method is that a Function is called directly with its name but a Method is called on objects only. Let's see all the important dictionary methods and functions one by one with examples.
copy() Method
The copy() method copies all the key:value pairs from an existing dictionary to a new dictionary.
Example
emp1 = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
emp2=emp1.copy()
print(emp2)
Output
{'id': 'e156', 'name': 'Allen', 'age': 34, 'salary': 15000}
clear() Method
The clear() method removes all the key:value pairs from an existing dictionary. After removing all the key:value pairs the dictionary becomes an empty dictionary.
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
emp.clear()
print(emp)
Output
{}
pop() Method
The pop() method remove and returns the value of a given key from an existing dictionary. Raises KeyError if the key is not found.
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
x=emp.pop('salary')
print('Removed salary',x,'from the dictionary')
print(emp)
Output
Removed salary 15000 from the dictionary {'id': 'e156', 'name': 'Allen', 'age': 34}
get() Method
The get() returns the value of a given key from an existing dictionary. Return None if the key is not found.
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
print(emp)
x=emp.get('name')
print('Name=',x)
print('City=',emp.get('city'))
Output
{'id': 'e156', 'name': 'Allen', 'age': 34, 'salary': 15000} Name= Allen City= None
items() Method
The items() method returns an object that contains all the (key, value) pairs of a dictionary. The pairs are stored as tuples in the object.
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
data=emp.items()
for x in data:
print(x)
Output
('id', 'e156') ('name', 'Allen') ('age', 34) ('salary', 15000)
keys() Method
The keys() method returns the sequence of keys from a dictionary.
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
print(emp.keys())
Output
dict_keys(['id', 'name', 'age', 'salary'])
values() Method
The values() method returns the sequence of values from a dictionary.
Example 1
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
print(emp.values())
Output
dict_values(['e156', 'Allen', 34, 15000])
Example 2
Check if a particular value exist in a dictionary or not.
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
if ('Allen' in emp.values())==True:
print('Allen exist in the dictionary')
else:
print('Allen does not exist in the dictionary')
Output
Allen exist in the dictionary
update() Method
The update() method merge key:value pairs from a new dictionary to an existing dictionary by adding or updating the elements as needed.
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
data={'post':'Engineer','city':'California','age':42}
emp.update(data)
print(emp)
Output
{'id': 'e156', 'name': 'Allen', 'age': 42, 'salary': 15000, 'post': 'Engineer', 'city': 'California'}
len() Function
The len() function returns the total number of key:value pairs present in a dictionary.
Example
emp = {'id':'e156', 'name':'Allen', 'age':34, 'salary':15000}
print(emp)
print('Length=',len(emp))
Output
{'id': 'e156', 'name': 'Allen', 'age': 34, 'salary': 15000} Length= 4
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
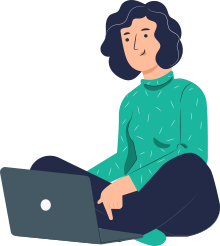