Naming Convention in Python Programming
Python Basic Concepts
In this lesson, we will learn about the Naming Convention in the Python programming language. We will go through the rules of naming Variables, Packages, Modules, Functions, and Classes, along with some examples and a quiz on it.
What is Naming Conventions
In Python programming, naming conventions are set of rules for choosing the valid name to be used for variable, package, module, class and function in a python program.
Naming Conventions rules for Variables, Packages, Modules and Functions (Methods) are:
- It should begin with an alphabet.
- It should be written in lowercase.
- There may be more than one alphabet, but without any spaces between them.
- Digits may be used but only after alphabet.
- No special symbol can be used except the underscore (_) symbol. When multiple words are needed, an underscore should separate them.
- No keywords or command can be used.
- All statements in Python language are case sensitive. Thus a name A (in uppercase) is considered different from a name a (in lowercase).
Now let's see some examples for more understanding.
Example 1
It should begin with an alphabet.
x # x is a valid variable name because it starts with an alphabet x
Example 2
There may be more than one alphabet, but without any spaces between them.
total # total is a valid variable name as there is no space between alphabets
Example 3
Digits may be used but only after alphabet.
ar15 # ar15 is a valid variable name as digits have been used after the alphabet
a6b2 # a6b2 is a valid variable name as digits have been used after the alphabet
Example 4
No special symbol should be present within the variable name except underscore _.
total_cost # total_cost is a valid variable name as there is an underscore
total cost # total cost is an invalid variable name as there is a space
total-cost # total-cost is an invalid variable name as there is a hyphen
total$ # total$ is an invalid variable name as there is a dollar symbol
Example 5
No keywords or command can be used as a variable name.
for # here for is an invalid variable name because it is a keyword in Python
if # if is an invalid variable name because it is a keyword in Python
elif # elif is an invalid variable name because it is a keyword in Python
while # while is an invalid variable name because it is a keyword in Python
Example 6
All statements in Python language are case sensitive. Thus a variable A (in uppercase) is considered different from a variable declared a (in lowercase).
a # a is a valid variable written in lowercase
A # A is a valid variable written in uppercase so both are different variables
Naming Conventions rules for Classes are:
- It should begin with an uppercase letter.
- There may be more than one alphabet, but without any spaces between them.
- No special symbol can be used.
- No keywords or command can be used as a class or interface name.
- If the class name is more than one word then the first letter of each word should be written in uppercase and the rest of the letter should be written in lowercase.
Example
Game # it is a valid 1 word class name
EmployeeInfo # it is a valid 2 words class name
TotalCostPrice # it is a valid 3 words class name
ServerNotActiveException # it is a valid 4 words class name
Note: Python’s built-in classes are typically lowercase words. But user defined classes should follow the above naming rules.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
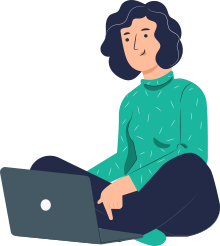