Sets in Python Programming
Data Structures in Python
In this lesson, we will understand what is Sets in Python Programming along with some examples.
What is Sets in Python?
A Set is an unordered collection of unique elements. We cannot add a duplicate element in a set.
A Set can contain elements of different data types. We can only add immutable elements in a set, we cannot update or change an element in a set, but we can add or remove elements from it.
Sets are mutable we can add or remove elements in a set once it is created.
Sets are useful when we are working with set related problems in mathematics like union, intersection, difference or symmetric difference, etc.
Declaration Syntax of a Set in Python
set_name = {elements1,element2,...}
Example
a = {5,8,6,2,9} # A set with five elements in it
Creating an Integer Type Set
A python program to show how to create and display an integer type set in python.
Example
a={15,2,49,51,34}
# printing the elements from an integer type set
for x in a:
print(x)
Output
2 34 15 49 51
Note: We can see that the elements are not displayed in order as it is store in the set. It proves that sets are unordered, as stated in its definition.
Creating a Floating Point Number Type Set
A python program to show how to create and display a floating point number type set in python.
Example
a={18.23,24.11,564.2369,44.17,98.513}
# printing the elements from a floating point number type set
for x in a:
print(x)
Output
44.17 18.23 98.513 564.2369 24.11
Creating a Character Type Set
A python program to show how to create and display a character type set in python.
Example
a={'a','e','i','o','u'}
# printing the elements from a character type set
for x in a:
print(x)
Output
o a i u e
Creating a String Type Set
A python program to show how to create and display a string type set in python.
Example
a={'apple','ball','cat','dog','eagle'}
# printing the elements from a string type set
for x in a:
print(x)
Output
apple eagle ball cat dog
Creating a Set of Different Types of Elements
A python program to show how to create and display a set of different types of elements in python.
Example
a={15,'Henry','2A',56,81,74,70.33,'B'}
# printing the elements from a set having different types of elements
for x in a:
print(x)
Output
74 70.33 15 81 Henry 2A 56 B
Create Sets from Existing Sequences
A python program to show how to create sets from existing sequences. A sequence can be any kind of sequence including string, tuples and lists.
Example
str='Python' # A String
name=['Allen','Peter','Henry','Stephen'] # A List
t=(12,89,63) # A Tuple
S1=set(str) # Creating a set from a String
S2=set(name) # Creating a set from another List
S3=set(t) # Creating a set from a Tuple
print(S1)
print(S2)
print(S3)
Output
{'t', 'n', 'y', 'o', 'h', 'P'} {'Henry', 'Allen', 'Peter', 'Stephen'} {89, 12, 63}
Creating an Empty Set
We can also create an empty set that does not contain elements at the time of declaration. See the example given below.
Example
a = set() # An empty set
print(a)
Output
set()
Use of Membership Operator in Set
Both in and not in operators works on Set just like they work for other sequences such as strings, lists and tuples. The in operator tells if an element is present in the set or not and not in does the opposite. See the example given below.
Example
a={15,2,49,51,86}
print(a)
if (49 in a) == True:
print('49 is prenest in the set')
else:
print('49 is not prenest in the set')
if (70 not in a) == True:
print('70 is not prenest in the set')
else:
print('70 is prenest in the set')
Output
{2, 15, 49, 51, 86} 49 is prenest in the set 70 is not prenest in the set
Set Methods
Set methods in Python are a collection of useful functions used to perform a specific task on a set. Let's see all the important set methods one by one with examples.
add() Method
The add() method adds an element to an existing set. It does not add the element if the element is already present in the set.
Example
a={11,9,26,34,53}
print('Original Set: ',a)
a.add(88)
print('Set after adding 88: ',a)
Output
Original Set: {34, 9, 11, 53, 26} Set after adding 88: {34, 9, 11, 53, 88, 26}
discard() Method
The discard() method removes an element from an existing set if it is a member element. It does nothing if the element is not a member element.
Example
a={11,9,26,34,53}
print('Original Set: ',a)
a.discard(34)
print('Set after removing 34: ',a)
Output
Original Set: {34, 9, 11, 53, 26} Set after removing 34: {9, 11, 53, 26}
clear() Method
The clear() method removes all the elements from an existing set. After removing all the elements the set becomes an empty set.
Example
a={11,9,26,34,53}
print('Original Set: ',a)
a.clear()
print('Set a after removing all the elements: ',a)
Output
Original Set: {34, 9, 11, 53, 26} Set a after removing all the elements: set()
pop() Method
The pop() method remove and returns an element from an existing set based on random choice. Raises KeyError if the set is empty.
Example
a={11,9,26,34,53}
print('Original Set: ',a)
x=a.pop()
print("Element removed: ",x)
print('Set a after removing the element: ',a)
Output
Original Set: {34, 9, 11, 53, 26} Element removed: 34 Set a after removing the element: {9, 11, 53, 26}
copy() Method
The copy() method returns a shallow copy of an existing set. A shallow copy means an exact copy of a set.
Example
a={11,9,26,34,53}
print('Original Set: ',a)
b=a.copy()
print("Shallow Copy Set: ",b)
Output
Original Set: {34, 9, 11, 53, 26} Shallow Copy Set: {34, 53, 9, 26, 11}
union() Method
The union() method returns the union of sets as a new set. The union of two or more sets is a set that contains all the elements present either in set A or set B.
Example
a={2,1,3,5,4}
b={5,7,6,8,1}
c={94,'Allen',75}
d={'Peter','Allen','Sam','Albert'}
print('Set a: ',a)
print('Set b: ',b)
print('Set c: ',c)
print('Set d: ',d)
e=a.union(b)
print('Set e (Union of Set a and b): ',e)
f=b.union(c,d)
print('Set f (Union of Set b, c and d): ',f)
Output
Set a: {1, 2, 3, 4, 5} Set b: {1, 5, 6, 7, 8} Set c: {'Allen', 75, 94} Set d: {'Allen', 'Sam', 'Peter', 'Albert'} Set e (Union of Set a and b): {1, 2, 3, 4, 5, 6, 7, 8} Set f (Union of Set b, c and d): {1, 'Sam', 'Peter', 5, 6, 7, 8, 75, 'Allen', 'Albert', 94}
update() Method
The update() method add elements from other specified sequences like (sets, lists, tuples) which are not present in the set that is to be updated.
Example
a={2,1,3,5,4}
b=[94,'Allen',75,1,4]
c=(56,89,34,75)
print('Set a: ',a)
print('List b: ',b)
print('Tuple c: ',c)
a.update(b,c)
print('Set a after update: ',a)
Output
Set a: {1, 2, 3, 4, 5} List b: [94, 'Allen', 75, 1, 4] Tuple c: (56, 89, 34, 75) Set a after update: {1, 2, 3, 4, 5, 34, 'Allen', 75, 56, 89, 94}
intersection() Method
The intersection() method returns the intersection of two sets as a new set. The intersection set is a set that contains the common elements present in both sets.
Example
a={2,1,3,5,4}
b={94,'Allen',75,1,4}
print('Set a: ',a)
print('Set b: ',b)
c=a.intersection(b)
print('Set c (intersection set): ',c)
Output
Set a: {1, 2, 3, 4, 5} Set b: {1, 4, 'Allen', 75, 94} Set c (intersection set): {1, 4}
intersection_update() Method
The intersection_update() method updates a set with the intersection of itself and another specified sets.
Example
a={2,1,3,5,4}
b={94,'Allen',75,10,17,4}
c={75,4,'Sam',17}
print('Set a: ',a)
print('Set b: ',b)
print('Set c: ',c)
a.intersection_update(b,c)
print('Set a after intersection update from Set a,b,c: ',a)
Output
Set a: {1, 2, 3, 4, 5} Set b: {4, 10, 75, 'Allen', 17, 94} Set c: {'Sam', 75, 4, 17} Set a after intersection update from Set a,b,c: {4}
Note: Here set a is updated with the value 4 which is the intersection (common) value in all the three sets a,b and c.
difference() Method
The difference() method returns the difference between two or more sets as a new set. The new set contains all elements that are in this set but not the others.
Example
a={2,1,3,5,4}
b={94,'Allen',75,10,17}
c={75,4,'Sam',17}
print('Set a: ',a)
print('Set b: ',b)
print('Set c: ',c)
d=a.difference(b,c)
print('Difference: Elements present in Set a but not in Set b or c: ',d)
Output
Set a: {1, 2, 3, 4, 5} Set b: {10, 75, 17, 94, 'Allen'} Set c: {17, 'Sam', 75, 4} Difference: Elements present in Set a but not in Set b or c: {1, 2, 3, 5}
difference_update() Method
The difference_update() method remove elements from this set which is also present in other sets.
Example
a={2,1,3,5,4}
b={94,'Allen',75,10,5}
c={75,4,'Sam',5}
print('Set a: ',a)
print('Set b: ',b)
print('Set c: ',c)
a.difference_update(b,c)
print('Set a after difference update from Set b and c: ',a)
Output
Set a: {1, 2, 3, 4, 5} Set b: {5, 'Allen', 10, 75, 94} Set c: {75, 4, 5, 'Sam'} Set a after difference update from Set b and c: {1, 2, 3}
symmetric_difference() Method
The symmetric_difference() method returns the symmetric difference of two sets as a new set. The method takes only one set as an arguments. The Symmetric Difference of two sets A and B is calculated as (Set A - Set B) union (Set B - Set A). In other words, it returns a new set having all elements that are present either in Set A or Set B but not both.
Example
a={1,2,3,4,5,6,7}
b={1,3,5,6,7,8,9}
print('Set a: ',a)
print('Set b: ',b)
c=a.symmetric_difference(b)
print('Set c after symmetric difference of Set a and b: ',c)
Output
Set a: {1, 2, 3, 4, 5, 6, 7} Set b: {1, 3, 5, 6, 7, 8, 9} Set c after symmetric difference of Set a and b: {2, 4, 8, 9}
Here Set a - Set b is {2, 4} and Set b - Set a is {8, 9} and the union of both the output is {2, 4, 8, 9}.
symmetric_difference_update() Method
The symmetric_difference_update() method updates a set with the symmetric difference of itself and another. In other words, it updates Set A with elements that are present either in Set A or Set B but not both.
Example
a={1,2,3,4,5,6,7}
b={1,3,5,6,7,8,9}
print('Set a: ',a)
print('Set b: ',b)
a.symmetric_difference_update(b)
print('Set a after symmetric difference update with Set b: ',a)
Output
Set a: {1, 2, 3, 4, 5, 6, 7} Set b: {1, 3, 5, 6, 7, 8, 9} Set a after symmetric difference update with Set b: {2, 4, 8, 9}
isdisjoint() Method
The isdisjoint() method returns True if two sets have a null intersection, which means no common elements exist in the sets.
Example
a={1,2,3,4}
b={5,6,7,8}
c={9,7,38,42}
print('Set a: ',a)
print('Set b: ',b)
print('Set c: ',c)
d=a.isdisjoint(b)
print('Is Set a and b are disjoint ',d)
e=b.isdisjoint(c)
print('Is Set b and c are disjoint ',e)
Output
Set a: {1, 2, 3, 4} Set b: {8, 5, 6, 7} Set c: {9, 42, 38, 7} Is Set a and b are disjoint True Is Set b and c are disjoint False
issuperset() Method
The issuperset() method returns True if all the elements of another set are present in this set. For example, Set A is a superset of another Set B if all the elements of Set B are elements of Set A also.
Example
a={1,2,3,4}
b={5,6,7,8,9,75,42,86,38}
c={9,7,38,42}
print('Set a: ',a)
print('Set b: ',b)
print('Set c: ',c)
d=b.issuperset(a)
print('Is all the elements of Set a are elements of Set b ',d)
e=b.issuperset(c)
print('Is all the elements of Set c are elements of Set b ',e)
Output
Set a: {1, 2, 3, 4} Set b: {5, 6, 7, 8, 9, 42, 75, 38, 86} Set c: {9, 42, 38, 7} Is all the elements of Set a are elements of Set b False Is all the elements of Set c are elements of Set b True
issubset() Method
The issubset() method returns True if all the elements of a particular set are present in another set. For example, Set A is a subset of another Set B if all the elements of Set A are elements of Set B also.
Example
a={1,2,3,4}
b={5,6,7,8,9,75,42,86,38}
c={9,7,38,42}
print('Set a: ',a)
print('Set b: ',b)
print('Set c: ',c)
d=c.issubset(b)
print('Is all the elements of Set c are elements of Set b ',d)
e=a.issubset(b)
print('Is all the elements of Set a are elements of Set b ',e)
Output
Set a: {1, 2, 3, 4} Set b: {5, 6, 7, 8, 9, 42, 75, 38, 86} Set c: {9, 42, 38, 7} Is all the elements of Set c are elements of Set b True Is all the elements of Set a are elements of Set b False
Functions to Process Sets
There are some useful functions available in python that we can apply on a set. The difference between Function and Method is that a Function is called directly with its name but a Method is called on objects only.
Let's see the functions given below with examples.
max() Function
The max() function returns the biggest element in a set.
Example
a={11,91,57,34,26,97,63,18}
print('Set: ',a)
print('Biggest element is :',max(a))
Output
Set: {97, 34, 11, 18, 57, 26, 91, 63} Biggest element is : 97
min() Function
The min() function returns the smallest element in a set.
Example
a={11,9,57,34,5,97,63,10}
print('Set: ',a)
print('Smallest element is :',min(a))
Output
Set: {97, 34, 5, 9, 10, 11, 57, 63} Smallest element is : 5
len() Function
The len() function returns the total numbers of elements present in a set.
Example
a={11,91,57,34,26,97,63,18}
print('Set: ',a)
print('Total number of elements :',len(a))
Output
Set: {97, 34, 11, 18, 57, 26, 91, 63} Total number of elements : 8
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
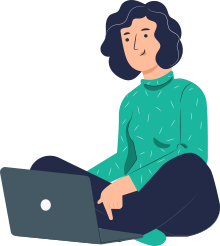