Lists in Python Programming
Data Structures in Python
In this lesson, we will understand what is List in Python Programming along with some examples.
What is List in Python?
A List in Python is a special type of variable that can store a sequence of different types of elements or items.
A list is similar to an array, but one major difference between list and array is that an array can store a sequence of elements of the same data type but, a list can store a sequence of elements of different data types. Hence lists are more versatile and useful than an array.
Like an array, a list can also increase or decrease their size dynamically whenever elements are added or removed from the list.
Lists are mutable. It means that we can modify the elements of a list directly.
Declaration Syntax of a List in Python
list_name = [elements1,element2,...]
Example
a = [5,8,6,2,9] # A list with five elements in it
Creating an Integer Type List
A python program to show how to create and display an integer type list in python.
Example
a=[15,2,49,51,86]
# printing the elements from an integer type list
for x in a:
print(x)
Output
15 2 49 51 86
Creating a Floating Point Number Type List
A python program to show how to create and display a floating point number type list in python.
Example
a=[18.23,24.11,564.2369,44.17,98.513]
# printing the elements from a floating point number type list
for x in a:
print(x)
Output
18.23 24.11 564.2369 44.17 98.513
Creating a Character Type List
A python program to show how to create and display a character type list in python.
Example
a=['a','e','i','o','u']
# printing the elements from a character type list
for x in a:
print(x)
Output
a e i o u
Creating a String Type List
A python program to show how to create and display a string type list in python.
Example
a=['apple','ball','cat','dog','eagle']
# printing the elements from a string type list
for x in a:
print(x)
Output
apple ball cat dog eagle
Creating a List of Different Types of Elements
A python program to show how to create and display a list of different types of elements in python.
Example
a=[15,'Henry','2A',56,81,74,70.33,'B']
# printing the elements from a list having different types of elements
for x in a:
print(x)
Output
15 Henry 2A 56 81 74 70.33 B
Create Lists from Existing Sequences
A python program to show how to create lists from existing sequences. A sequence can be any kind of sequence including string, tuples and lists.
Example
str='Python' # A String
name=['Allen','Peter','Henry','Stephen'] # A List
t=(12,89,63) # A Tuple
L1=list(str) # Creating a list from a String
L2=list(name) # Creating a list from another List
L3=list(t) # Creating a list from a Tuple
print(L1)
print(L2)
print(L3)
Output
['P', 'y', 't', 'h', 'o', 'n'] ['Allen', 'Peter', 'Henry', 'Stephen'] [12, 89, 63]
Creating an Empty List
We can also create an empty list that does not contain elements at the time of declaration. See the example given below.
Example
a = [] # An empty list
b = list() # Another way of creating an empty list
print(a)
print(b)
Output
[] []
List Slicing
List slicing is the process of accessing a part of the list using either positive or negative indexing with the help of list slicing syntax given below.
list_name[ start : end+1 : step]
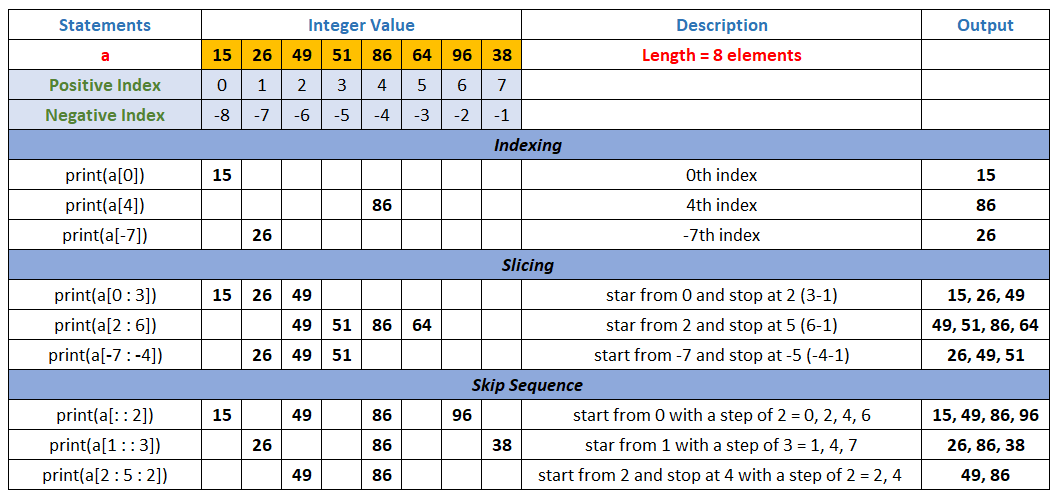
Example
a=[15,26,49,51,86,64,96,38]
print(a[0])
print(a[4])
print(a[-7])
print(a[0:3])
print(a[2:6])
print(a[-7:-4])
print(a[::2])
print(a[1::3])
print(a[2:5:2])
Output
15 86 26 [15, 26, 49] [49, 51, 86, 64] [26, 49, 51] [15, 49, 86, 96] [26, 86, 38] [49, 86]
Modifying Elements of a List
We can modify an element of a list just by assigning a new value to the element's index as shown below.
Example
a=[15,2,49,51,86]
a[0]=12
a[2]=37
# printing the list after modifying the elements at index 0 and 2
print(a)
Output
[12, 2, 37, 51, 86]
Joining Lists
We can use the concatenation operator + to join two lists and returns the concatenated list as shown below.
Example
a=[15,2,49,51,86]
b=[56,8,97,4]
c=a+b
print(a)
print(b)
print(c)
Output
[15, 2, 49, 51, 86] [56, 8, 97, 4] [15, 2, 49, 51, 86, 56, 8, 97, 4]
Use of Membership Operator in List
Both in and not in operators works on List just like they work for other sequences such as strings. The in operator tells if an element is present in the list or not and not in does the opposite. See the example given below.
Example
a=[15,2,49,51,86]
print(a)
if (49 in a) == True:
print('49 is prenest in the list')
else:
print('49 is not prenest in the list')
if (70 not in a) == True:
print('70 is not prenest in the list')
else:
print('70 is prenest in the list')
Output
[15, 2, 49, 51, 86] 49 is prenest in the list 70 is not prenest in the list
List Methods
List methods in Python are a collection of useful functions used to perform a specific task on a list. Let's see all the important list methods one by one with examples.
append() Method
The append() method adds an element at the end of an existing list.
Example
a=[11,9,26,34,53]
print('Original List: ',a)
a.append(88)
print('List after appending 88: ',a)
Output
Original List: [11, 9, 26, 34, 53] List after appending 88: [11, 9, 26, 34, 53, 88]
extend() Method
The extend() method appends another list at the end of an existing list.
Example
a=[15,2,49,51,86]
b=[9,7,5,62]
print('List a: ',a)
print('List b: ',b)
a.extend(b)
print('List a after appending list b: ',a)
Output
List a: [15, 2, 49, 51, 86] List b: [9, 7, 5, 62] List a after appending list b: [15, 2, 49, 51, 86, 9, 7, 5, 62]
count() Method
The count() method returns the number of occurrences of a specified element in an existing list.
Example
a=[11,9,26,34,26,84,97,26]
print('Original List: ',a)
print('Total occurrence of element 26: ',a.count(26))
Output
Original List: [11, 9, 26, 34, 26, 84, 97, 26] Total occurrence of element 26: 3
index() Method
The index() method returns the index of the first occurrence of the specified element in an existing list. The index() method raise ValueError if the element is not found.
Example
a=[11,9,26,34,26,84,97,26]
print('Original List: ',a)
print('Index of first occurrence of element 26: ',a.index(26))
Output
Original List: [11, 9, 26, 34, 26, 84, 97, 26] Index of first occurrence of element 26: 2
insert() Method
The insert() method inserts an element at a specific index in an existing list.
Example
a=[11,9,26,34,84]
print('Original List: ',a)
a.insert(2, 59)
print('List after inserting 59 at index 2: ',a)
Output
Original List: [11, 9, 26, 34, 84] List after inserting 59 at index 2: [11, 9, 59, 26, 34, 84]
pop() Method
The pop() method removes and returns the last element of a list if the element's index is not specified. To remove a specific element from the list, we have to specify its index.
Example
a=[11,9,26,34,84]
print('Original List: ',a)
a.pop()
print('List after removing the last element: ',a)
a.pop(2)
print('List after removing the element at index 2: ',a)
Output
Original List: [11, 9, 26, 34, 84] List after removing the last element: [11, 9, 26, 34] List after removing the element at index 2: [11, 9, 34]
remove() Method
The remove() method removes the first occurrence of the specified element in an existing list. The remove() method raise ValueError if the element is not found.
Example
a=[11,9,26,34,26,84,97,26]
print('Original List: ',a)
a.remove(26)
print('List after removing the first occurrence of element 26: ',a)
Output
Original List: [11, 9, 26, 34, 26, 84, 97, 26] List after removing the first occurrence of element 26: [11, 9, 34, 26, 84, 97, 26]
clear() Method
The clear() method removes all the elements from an existing list and the list becomes an empty list.
Example
a=[11,9,26,34,26,84,97,26]
print('Original List: ',a)
a.clear()
print('List after removing all the elements: ',a)
Output
Original List: [11, 9, 26, 34, 26, 84, 97, 26] List after removing all the elements: []
reverse() Method
The reverse() method reverses the order of element in an existing list.
Example
a=[11,9,26,34,84,97]
print('Original List: ',a)
a.reverse()
print('List after reversing the order of elements: ',a)
Output
Original List: [11, 9, 26, 34, 84, 97] List after reversing the order of elements: [97, 84, 34, 26, 9, 11]
sort() Method
The sort() method sorts the elements of a list in increasing order by default. To sort the list in decreasing order we have use sort(reverse=True). See the example given below.
Example
a=[11,9,26,1,84,5]
print('Original List: ',a)
a.sort()
print('List after sorting the elements in increasing order: ',a)
a.sort(reverse=True)
print('List after sorting the elements in decreasing order: ',a)
Output
Original List: [11, 9, 26, 1, 84, 5] List after sorting the elements in increasing order: [1, 5, 9, 11, 26, 84] List after sorting the elements in decreasing order: [84, 26, 11, 9, 5, 1]
Functions to Process Lists
There are some useful functions available in python that we can apply on a list. The difference between Function and Method is that a Function is called directly with its name but a Method is called on objects only.
Let's see the functions given below with examples.
max() Function
The max() function returns the biggest element in a list.
Example
a=[11,91,57,34,26,97,63,18]
print('List: ',a)
print('Biggest element is :',max(a))
Output
List: [11, 91, 57, 34, 26, 97, 63, 18] Biggest element is : 97
min() Function
The min() function returns the smallest element in a list.
Example
a=[11,9,57,34,5,97,63,10]
print('List: ',a)
print('Smallest element is :',min(a))
Output
List: [11, 9, 57, 34, 5, 97, 63, 10] Smallest element is : 5
len() Function
The len() function returns the total numbers of elements present in a list.
Example
a=[11,91,57,34,26,97,63,18]
print('List: ',a)
print('Total number of elements :',len(a))
Output
List: [11, 91, 57, 34, 26, 97, 63, 18] Total number of elements : 8
Iterate all Elements in a List using range() Function
We can also iterate all elements in a list using range() function as shown below.
Example
a=[15,2,49,51,86]
# printing the elements from an integer type list
for i in range(0,len(a)):
print(a[i])
Output
15 2 49 51 86
Create an Integer List from User Input
A python program to show how to create an integer list from user input.
Example
a=list()
print('Enter 5 numbers')
for i in range(5):
a.append(int(input()))
print(a)
Output
Enter 5 numbers 12 8 47 6 91 [12, 8, 47, 6, 91]
Nested List
A list within another list is known as Nested List. We can use nested list to create matrix in python. See the examples of nested list given below.
Example 1
A python program to show how to create a nested list to store the roll and names and age of three candidates.
a=[[1,'Allen',15],[2,'Peter',17],[3,'Stephen',16]]
# printing the entire list
print(a,'\n')
# printing the list row by row
for r in range(3):
for c in range(3):
print(a[r][c],end=' ')
print()
Output
[[1, 'Allen', 15], [2, 'Peter', 17], [3, 'Stephen', 16]] 1 Allen 15 2 Peter 17 3 Stephen 16
We can assume the above nested list as shown in the image below.
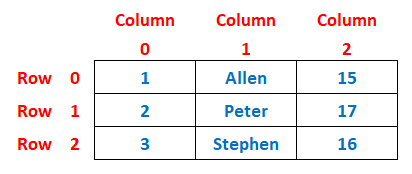
We can access any cell of a nested list using the following syntax.
list_name = [row_index][column_index]
In the above program, we have run a nested for loop to access all the elements of the nested list. When the value of outer for loop r is 0, then the inner for loop c runs from 0 to 2 and prints all the elements of the first row of the nested list.
When the value of outer for loop r is 1, then again the inner for loop c runs from 0 to 2 and prints all the elements of the second row of the nested list and in the same way it prints the third row also.
Example 2
A python program to store numbers in a 3x3 (3 rows and 3 columns) integer matrix, print the matrix and find the sum of all numbers in it.
a=[[],[],[]]
sum=0
# enter numbers in a 3x3 matrix
print('Enter 9 numbers')
for r in range(3):
for c in range(3):
a[r].append(int(input()))
# printing the matrix
for r in range(3):
for c in range(3):
print(a[r][c],end=' ')
sum=sum+a[r][c]
print()
print('Sum of all the numbers =',sum)
Output
Enter 9 numbers 12 18 45 63 20 47 33 84 96 12 18 45 63 20 47 33 84 96 Sum of all the numbers = 418
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
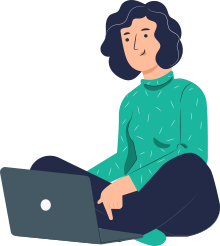