Variable in Python Programming with Example
Python Basic Concepts
In this lesson, we will learn about the variable in Python programming and its application, along with an example.
Definition of Variable in Python
A variable in Python is just a human-readable name used to store data in computer memory. A variable is also used in a computer program to store, read and change the data in computer memory.
A variable is also known as Identifier because it is used for identifying values in a computer program.
When you declare a variable in Python programming language, it means that you are asking the operating system to reserve a piece of memory with that variable name for storing data.
What is data?
Data is a collection of facts or figure or combination of both (facts and figure).
- Facts - Any word that is consist of only alphabets is known as Facts. Example: john, mango, book, etc these words are consist of only alphabets. So each of them is a data but consist of Facts.
- Figure - Any number whether it is whole number or real number is a Figure. Example: 15, 84.13, 5486, etc. So each of them is a data but consist of Figure.
- Combination of Both (Facts and Figure) - Anything which is consist of Facts and Figure is known as Alphanumeric Text. Example: 13street, alpha56, dx14c5, etc. So each of them is a data but consist of Alphanumeric Text.
Unlike other programming languages like C, C++ or Java, Python has no command for declaring a variable. A variable is created in python the moment you assign a value to it.
Let's see some examples for more understanding.
Example 1
To declare an integer and float variable in python.
x=5
x=4.23
Here you can see that we have declared a variable x and assigned the value 5 in it so, it becomes an integer variable. We can use the same variable to store another type of value in it as you can see, on line number 2 we have used the same variable name x to store a float value 4.23 in it so, now the variable x becomes a float variable.
Example 2
To declare a string variable in python.
y="apple"
Here you can see that we have declared a variable y and assigned a string value apple in it so, it becomes a string variable.
Example 3
To declare a boolean variable in python.
z=True
Here you can see that we have declared a variable z and assigned a boolean value True in it so, it becomes a boolean variable.
Example 4
To declare multiple variables and assign multiple values in a single line.
a, b, c, d = 5, 4.26, "apple", True
a=5; b=4.26; c="apple"; d=True
Here you can see that we have declared 4 variables a, b, c, d and assigned values 5, 4.26, "apple", True respectively in a single line in two different ways, one using comma (,) and another using semicolon (;).
You can use any one method that you like. So the value of a is 5, value of b is 4.23, value of c is apple and value of d is True which becomes a boolean variable.
Example 5
To declare same value in multiple variables in a single line.
a=b=c=15
Here you can see that we have declared 3 variables a, b, c and assigned the same value 15 in all the variables respectively in a single line.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
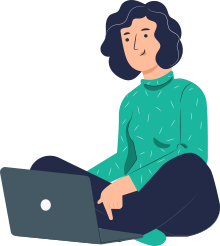