for Loop in Python Programming
Loops in Python
In this lesson, we will learn what is for loop, and how many ways a for loop can be used.
What is for Loop
A for loop in Python is a repetition control structure which allows us to write a loop that is executed a specific number of times. Python for loop can be used in a list, tuple, set, string, etc.
Syntax of for Loop
for x in sequence:
# body of the for loop
Here x is a variable which takes values from a sequence. A sequence can be a tuple, set, string or range() function.
Let’s see some examples for more understanding.
Example 1
# List of numbers
num=[10,12,8,25,82,64]
for x in num:
print(x)
Output
10 12 8 25 82 64
In the above example, we have run a for loop. Each time when the loop runs, it reads a number from the num list in variable x one by one, and then we print those numbers on the screen using the print() function.
Example 2
fruit='Pineapple'
for x in fruit:
print(x)
Output
P i n e a p p l e
In the above example, we have run a for loop. Each time when the loop runs, it reads a character from the string variable fruit in variable x one by one, and then we print those characters on the screen using the print() function.
Use of range() Function in for Loop
Python range() function is used to generate a sequence of numbers. It is commonly used in Python for loop and Python while loop. The range() function accept only integer value as its parameters.
Syntax of range()
range(start, stop, step)
- start - The start value of the Python range() function is 0 by default if not specified.
- stop - The stop value of the Python range() function is the number before which the sequence of numbers will generate. The last value of the number's sequence is always stopped value -1. For example range(1, 4) will generate number as 1, 2, and 3.
- step - The step value of the Python range() function specifies the increment or decrement order of the generated numbers. By default the step value is 1 if not specified. For example range(1, 10, 2) will generate number as 1, 3, 5, 7 and 9 with an increment value of 2 between the numbers.
Let’s see some examples for more understanding.
Example 1
Python for loop program to print the numbers from 1 to 10 on the screen using range() function.
for x in range(1, 11):
print(x)
Output
1 2 3 4 5 6 7 8 9 10
In the above example, we have run a for loop from 1 to 11 using the range() function. Each time when the loop runs, we print the value of the variable x on the screen on a separate line using the print() function. The loop ends when the value of x is more than 10.
Example 2
Python for loop program to print all the even numbers from 10 to 20 on the screen using range() function.
for x in range(10, 21, 2):
print(x)
Output
10 12 14 16 18 20
In the above example, we have run a for loop from 10 to 21 using the range() function. Each time when the loop runs, we print the value of the variable x on the screen on a separate line using the print() function and then the value of x is incremented by 2. The loop ends when the value of x is more than 20.
Example 3
Python for loop program to print all the numbers from 10 to 1 in reverse order on the screen using range() function.
for x in range(10, 0, -1):
print(x)
Output
10 9 8 7 6 5 4 3 2 1
In the above example, we have run a for loop from 10 to 0 in reverse order using range() function. Each time when the loop runs, we print the value of the variable x on the screen on a separate line using the print() function and then the value of x is decremented by -1. The loop ends when the value of x is less than 1.
Nested for Loop
Python programming allows using one for loop inside another for loop. This is known as nested for loop.
Syntax of Nested for Loop
for x in sequence:
for y in sequence:
Example
for x in range(1,6):
for y in range(1, x+1):
print(y,end='')
print()
Output
1 12 123 1234 12345
In the above example, we have run two loops, one outer loop and another inner loop. The outer loop runs from 1 to 5, and the inner loop runs from 1 to the current value of the outer loop + 1 (for example, when the value of x is 1, the inner loop runs from 1 to 2. When the value of x is 2, the inner loop runs from 1 to 3 and so on). The program ends when the value of x is more than 5.
Python Infinite for Loop
Python infinite for loop is a loop that never ends. It keeps on executing unless and until we terminate the loop using the break statement. In Python, we need to import the itertools module to use an infinite loop.
Syntax of Infinite for Loop in Python
import itertools
for x in itertools.repeat(1):
# body of the infinite for loop
Example
import itertools
i=0
for x in itertools.repeat(1):
print('Hello World')
i=i+1
if i==5:
break # terminate the infinite loop when the value of i is 5
Output
Hello World Hello World Hello World Hello World Hello World
In the above example, we have run an infinite for loop using the itertools.repeat(1) statement. This statement will repeat the value of x to 1 always, which results in an infinite for loop that never ends. To terminate an infinite for loop we have to use a break statement as shown above in the example.
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
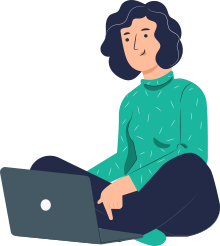