Typecasting in Python Programming Language
Python Basic Concepts
In this lesson, we will learn about what is Typecasting and different types of it. We will also go through some examples and take a quiz at the end.
What is Typecasting
In Python the process of changing one data type into another data type is known as Typecasting. There are two types of typecasting: Implicit and Explicit.
- Implicit Typecasting - This is also known as automatic typecasting. In this type of casting (conversion) the data is converted from one data type to another data automatically without programmer interference.
- Explicit Typecasting - In this type of casting (conversion) the data is converted from one data type to another data type by the programmer itself.
Now let's see the examples for more understanding.
Example 1 (Implicit Typecasting)
a = 5
b = 2.5
c = a+b # result of c will be 7.5
Here you can see that the 1st variable a is an integer variable because its value is a whole number without any decimal point in it. Whereas the 2nd variable b is a float variable because it contains a number having decimal point in it.
Now the 3rd variable c stores the sum of the values of 1st and 2nd variables which is 7.5 so, the 3rd variable becomes float variable because the sum is a decimal number. This type of conversion is known as Implicit Casting.
Example 2 (Explicit Typecasting from integer to float using float() function)
a = 8
b = float(a) # result of b will be 8.0
Here you can see that we have changed the integer value 8 into float value 8.0 using the float() function.
Example 3 (Explicit Typecasting from float to integer using int() function)
a = 5.8
b = int(a) # result of b will be 5
Here you can see that we have changed the float value 5.8 into integer value 5 using the int() function.
Example 4 (Explicit Typecasting from integer to string using str() function)
a = 456
b = str(a) # result of b will be 456 but it will be treated as string.
Here you can see that we have changed the integer value 456 into string value "456" using the str() function.
Example 5 (Explicit Typecasting from string to integer using int() function)
a = "18"
b = int(a) # result of b will be 18 and it will be an integer type value.
Here you can see that we have changed the string value "18" into integer value 18 using the int() function.
Example 6 (Explicit Typecasting from float to string using str() function)
a = 24.19
b = str(a) # result of b will be 24.19 but it will be treated as string.
Here you can see that we have changed the float value 24.19 into string value "24.19" using the str() function.
Example 7 (Explicit Typecasting from string to float using float() function)
a = "57.49"
b = float(a) # result of b will be 57.49 and it will be a float type value.
Here you can see that we have changed the string value "57.49" into float value 57.49 using the float() function.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
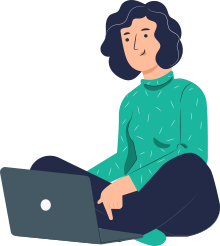