Membership Operators in Python
Operators in Python
In this lesson, we will learn what is the Membership Operators and how it works in Python programming with some examples.
What is Membership Operators
Membership Operators are used in Python to check whether a value or variable is found in a sequence (string, list, tuple, set and dictionary). But remember that in a dictionary, we can only check for the presence of a key, not the value.
There are 2 types of membership operators in Python and they are:
- in
- not in
Now let's see the examples of the membership operators one by one for more understanding.
in Operator
in operator is used to check if value or variable is found in the sequence. If found then the result will be True otherwise the result will be False.
Example
a="I am learning Python"
b={1:'apple',2:'ball'} # it is a dictionary where 1 is key and apple is its value
x="Python" in a # output of x is True
y=3 in b # output of y is False
In the example above the output of x is True because the string Python is present in the variable a. Whereas the output of y is False because the key 3 is not present in the dictionary b.
not in Operator
not in operator is used to check if value or variable is not found in the sequence. If not found then the result will be True otherwise the result will be False.
Example
a="I am learning Python"
b={1:'apple',2:'ball'} # it is a dictionary where 1 is key and apple is its value
x="Hello" not in a # output of x is True
y=1 not in b # output of y is False
In the example above the output of x is True because the string Hello is not present in the variable a. Whereas the output of y is False because the key 1 is present in the dictionary b.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
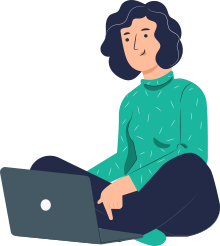