Text File Handling in Python
File Handling in Python
In this lesson, we will understand what Text File is and how to create and handle them in Python Programming, along with some examples.
What is Text File Handling
Text File Handling is a process in which we create a text file and store data permanently on a hard disk so that it can be retrieved from the memory later for use in a program.
In a text file, whatever data we store is treated as text. Even if we store numbers, it is treated as text.
Operation on Text File
There are different types of operations that we can perform on a text file, they are:
- Opening and Closing a Text File.
- Write to a file.
- Read from a file.
- Copy a file from one location to another.
- Rename a file.
- Delete a file.
Text File Opening Modes
Below we have discussed the different types of text file opening modes used to open a text file in python.
Mode | Description |
r | Open an existing file for reading only. The file pointer stands at the beginning of the file. |
r+ | Open file for both reading and writing. The file pointer stands at the beginning of the file. |
w | Open file for writing only. It creates the file if it does not exist. If the file exists, then it erases all the contents of the file. The file pointer stands at the beginning of the file. |
w+ | Open file for both reading and writing. It creates the file if it does not exist. If the file exists, then it erases all the contents of the file. The file pointer stands at the beginning of the file. |
a | Open file for appending text. Text is added to the end of the file. It creates the file if it does not exist. The file pointer stands at the end of the file. |
a+ | Open file for reading and appending text. Text is added to the end of the file. It creates the file if it does not exist. The file pointer stands at the end of the file. |
Open a text file
We use open() function to open a text file in python. The syntax is given below.
Syntax of open() function
file_object = open('file_name', 'access_mode')
In the above syntax, file_object is the variable's name in which the file handle will be stored as an object. The file_name is the name of the file along with its path. The access_mode is the mode which is used while opening a file..
Example
fileobj = open('D:/data.txt','w+')
In the above statement, the file data.txt is opened in reading and writing modes under D drive in the windows operating system. The file pointer will stand at the beginning of the file. That means we can write data, and at the same time, we can also read data from the file using the file object named fileobj.
Open a text file using with statement
In python, we can also open a text using a with statement. The syntax is given below.
Syntax of with statement
with open('file_name', 'access_mode') as file_object:
Example
with open('D:/data.txt','w+') as fileobj:
fileobj.write('Hello World')
The advantage of using a with statement while opening a file is that it ensures that the file will close automatically when the program comes out of the with block. This way the user don't have to close the file explicitly.
Close a file
We use the close() method to close a file in python. Any unsaved data is written to the file before it is closed.
Syntax of close() method
file_object.close():
Example
fileobj = open('D:/data.txt','w+')
fileobj.close()
Writing to a text file
To write data in a text file, first, we have to open the file either in write or append mode. If we open an existing file in write mode, the previous data will be erased, and the file pointer will stand at the beginning of the file.
If the file already exists, we will open the file in append mode so the file pointer will stand at the end, and we can add new data to the file. In append mode, the previous data is not erased.
There are two methods that we can use to write data in a text file, and they are:
- write()
- writelines()
write() Method
The write() method is used to write a string in an opened file. This method does not automatically add a newline character (\n) at the end of the string. We need to manually add the newline character (\n) at the end of the string to mark the end of the line.
Example
with open('D:/data.txt','w+') as fileobj:
print('Enter five animals names')
for i in range(5):
name=input('Enter the animal name: ')
fileobj.write(name)
fileobj.write('\n')
Output
Enter five animals names Enter the animal name: Dog Enter the animal name: Cat Enter the animal name: Mouse Enter the animal name: Elephant Enter the animal name: Horse
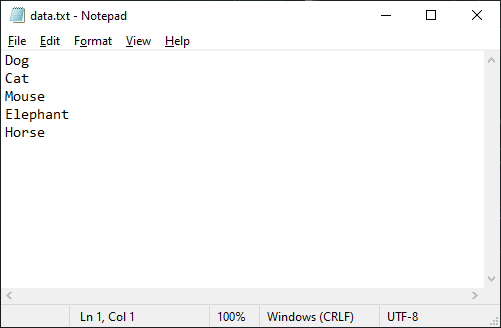
writelines() Method
The writelines() method is used to write a list of strings in an opened file. Like the write() method, this method does not automatically add a newline character (\n) at the end of the string. We need to manually add the newline character (\n) at the end of the string to mark the end of the line.
Example
with open('D:/countries.txt','w+') as fileobj:
print('Enter five countries names')
country = [] # An emplty list
for i in range(5):
name=input('Enter the country name: ')
country.append(name + '\n')
fileobj.writelines(country)
Output
Enter five countries names Enter the country name: America Enter the country name: India Enter the country name: Korea Enter the country name: Japan Enter the country name: Australia
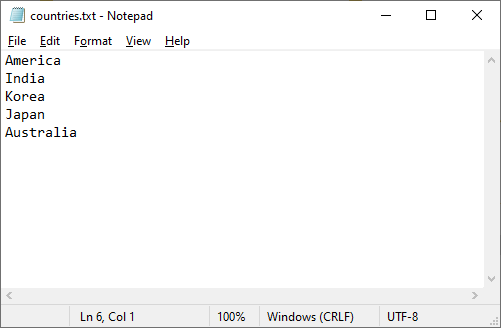
Reading from a text file
To read data from a text file, we have to open the file in read mode.
There are three methods that we can use to read data from a text file, and they are:
- read()
- readline(n)
- readlines()
read() Method
The read() method reads a specified number of bytes of data from a file. The entire file content is read if the number of bytes is not given.
Example
with open('D:/countries.txt','r') as fileobj:
# read 5 bytes from the file
data = fileobj.read(5)
print(data)
# read the entire content of the file
data = fileobj.read()
print(data)
Output
Ameri ca India Korea Japan Australia
In the above program, we first read 5 bytes (characters) from the file, so it reads up to Ameri, and the file pointer stands after i in the file. Later we read the entire file content, so it starts reading from ca to Australia.
readline(n) Method
The readline(n) method reads one complete line from a file that terminates with a newline (\n) character. It can also read a specified number of bytes from a file but ends reading when a newline (\n) character is found while reading the specified number of bytes.
Example
with open('D:/countries.txt','r') as fileobj:
# read the first line from the file
data = fileobj.readline()
print(data)
# read 10 bytes from the file
data = fileobj.readline(10)
print(data)
Output
America India
readlines() Method
The readlines() method reads all the lines from the file, including the newline (\n) character, and returns the lines as a list of strings.
Example
with open('D:/countries.txt','r') as fileobj:
# read all the lines from the file including the newline (\n) character
data = fileobj.readlines()
print(data)
Output
['America\n', 'India\n', 'Korea\n', 'Japan\n', 'Australia\n']
We can use the for loop to print all the lines on the screen. See the example given below.
Example
with open('D:/countries.txt','r') as fileobj:
# read all the lines from the file
data = fileobj.readlines()
# print all the lines on the screen using the for loop
for line in data:
print(line, end='')
Output
America India Korea Japan Australia
In the above program, the line is a variable that reads each element from the list data and prints it on the screen.
Setting file pointer (cursor)
To read a file randomly, we need to place the file pointer (cursor) in a file at the specified location so we can read characters from that location onwards. To do so, python provides two methods to work with the file pointer, and they are:
- tell()
- seek()
tell() Method
The tell() method returns an integer that specifies the position of the file pointer in a file. The position specifies the number of bytes the file pointer is standing at from the beginning of the file.
Example
with open('D:/countries.txt','r') as fileobj:
# read 5 bytes from the beginning of the file
data = fileobj.read(5)
print(data)
# print the file pointer position in the file
x = fileobj.tell()
print('The file pointer is standing at position %d in the file' %(x))
# read next byte from the file
data = fileobj.read(1)
print(data)
# print the file pointer position in the file
x = fileobj.tell()
print('The file pointer is standing at position %d in the file' %(x))
Output
Ameri The file pointer is standing at position 5 in the file c The file pointer is standing at position 6 in the file
After reading 5 bytes from the file in the above program, the file pointer stands at the 5th position (at i in the file). So the next byte in the file after i is c. When we read one more byte from the file, it reads the character c, and then the file pointer stands at position 6.
seek() Method
The seek() method sets the file pointer at a specific position in a file. Using the seek() method, we can move the file pointer in a file either forward or backwards. The syntax is given below.
Syntax of seek() method
fileobj.seek(offset, whence)
Here the offset is the number of bytes to move forward or backwards in the file from the whence arguments. The whence argument specifies from which position the file pointer should move forward or backwards in the file.
The whence argument provides the following values.
- 0 means moving the file pointer from the beginning of the file.
- 1 means moving the file pointer from the current position in file position.
- 2 means moving the file pointer from the end of the file.
The default value of the whence argument is 0, which means from the beginning of the file.
See the examples below to clearly understand how to implement the seek() method in file handling.
Move the file pointer to the beginning of the file
with open('D:/countries.txt','r') as fileobj:
# read 5 bytes from the beginning of the file
data = fileobj.read(5)
print(data)
# move file pointer to the beginning of the file
fileobj.seek(0)
# read a complete line from the beginning of the file
data = fileobj.readline()
print(data)
Output
Ameri America
Move the file pointer to the end of the file
with open('D:/countries.txt','r+') as fileobj:
# move file pointer to the end of the file
fileobj.seek(0,2)
# write canada at the end of the file
fileobj.write('Canada\n')
# move file pointer to the beginning of the file
fileobj.seek(0)
# read the complete content of the file
data = fileobj.read()
print(data)
Output
America India Korea Japan Australia Canada
Move the file pointer 10 bytes ahead from the beginning of the file
with open('D:/countries.txt','r') as fileobj:
# move the file pointer 10 bytes ahead from the beginning of the file
fileobj.seek(10)
# now read 8 bytes
data = fileobj.read(8)
print(data)
Output
ndia Kor
Move the file pointer backward from the current location in the file
with open('D:/countries.txt','r') as fileobj:
# read 36 bytes from the file
data = fileobj.read(36)
print(data)
# move the file pointer 11 bytes backward from the current location in the file
fileobj.seek(fileobj.tell()-11)
# now read the full content of the file from the current location onwards
data = fileobj.read()
print(data)
Output
America India Korea Japan Australia Australia Canada
Note: If the file is opened in non-binary mode (without using b in the access mode), then we can't move the file pointer backwards with a negative value from the current location in the file. If we do so, it will give the following error, as shown below.
Move the file pointer backwards with a negative value in a non-binary mode
with open('D:/countries.txt','r') as fileobj:
# read 36 bytes from the file
data = fileobj.read(36)
print(data)
# move the file pointer 11 bytes backward from the current location in the file
fileobj.seek(-11,1)
# now read the full content of the file from the current location onwards
data = fileobj.read()
print(data)
Output
Traceback (most recent call last): File "D:\python\example.py", line 7, infileobj.seek(-11,1) io.UnsupportedOperation: can't do nonzero cur-relative seeks
We have to open the file in binary mode to move the file pointer backwards with a negative value. See the example given below.
Move the file pointer backwards with a negative value in a binary mode
with open('D:/countries.txt','rb') as fileobj:
# read 36 bytes from the file
data = fileobj.read(36).decode('utf-8')
print(data)
# move the file pointer -11 bytes backward from the current location in the file
fileobj.seek(-11,1)
# now read the full content of the file from the current location onwards
data = fileobj.read().decode('utf-8')
print(data)
Output
America India Korea Japan Austra pan Australia Canada
The newline character in binary mode in the above program is \r\n. So 36 bytes will end at Austra, where \r\n is included at the end of each line. Now moving backwards, -11 bytes will move the file pointer to the pan of Japan. When we are reading the entire content of the file from the current location, this will print the content starting from pan to Canada.
The decode('utf-8') converts the text into readable format. Without it, the content will look as shown below.
Move the file pointer backwards with a negative value in a binary mode
with open('D:/countries.txt','rb') as fileobj:
# read 36 bytes from the file
data = fileobj.read(36)
print(data)
# move the file pointer -11 bytes backward from the current location in the file
fileobj.seek(-11,1)
# now read the full content of the file from the current location onwards
data = fileobj.read()
print(data)
Output
b'America\r\nIndia\r\nKorea\r\nJapan\r\nAustra' b'pan\r\nAustralia\r\nCanada\r\n'
In the above output, b at the beginning of each line represents the string into binary format, and \r\n represents the newline characters in the binary format.
Write multiple lines in a file
Let's write a program where we will create a file and write multiple lines in it.
Example
# create the file record.txt at D:/ drive
with open('D:/record.txt','a+') as fileobj:
# write some text in the file and press enter key twice to end writing
print('Write some text and press enter key twice to end writing')
data = input()
while len(data)>0:
fileobj.write(data+'\n')
data = input()
# move the file pointer to the beginning of the file
fileobj.seek(0)
# read the full content of the file
data = fileobj.read()
print(data)
Output
Write some text and press enter key twice to end writing We are learning text file handling in python. We have create a file record.txt and writing this text in it. We are learning text file handling in python. We have create a file record.txt and writing this text in it.
Copy a file from one location to another
To copy a file from one location to another, we need to use the shutil.copy() method. This method is available in the shutil module.
Example
import shutil
src_file = r'D:\countries.txt'
dest_file = r'E:\countries.txt'
shutil.copy(src_file, dest_file)
print('File Copied Successfully')
Output
File Copied Successfully
Rename a file
We need to use the os.rename() method to rename a file. This method is available in the os module.
Example
import os
old_name = r'D:\countries.txt'
new_name = r'D:\countries2.txt'
os.rename(old_name, new_name)
print('File Renamed Successfully')
Output
File Renamed Successfully
Delete a file
We need to use the os.remove() method to delete a file. This method is available in the os module.
Example
import os
file_name = r'D:\countries2.txt'
os.remove(file_name)
print('File Deleted Successfully')
Output
File Deleted Successfully