Function in Python Programming
Function in Python
In this lesson, we will understand what is Function in Python Programming along with some examples.
What is Function in Python?
A Function in python is a block of statements that is defined to perform a specific task. A function is also called a reusable code because once it is created, it can be used again and again.
To clearly understand a function let's take a real life example of a function.
In the early days, we used to wash our clothes manually by using our hands, and we used to repeat this task daily. But nowadays, we wash our clothes using a washing machine that does the same task that we used to do in our early days by hands. Here, the washing machine is a perfect example of a function, we can use it, again and again, to wash our clothes whenever required.
Note: In python a functions are objects. We will learn about objects later on in our OOPs in Python chapter.
Function Categories
In python, there are three categories of functions available, and they are:
- Built-in Functions : These functions are predefined, and we can use them any time we want in our python program. For example len(), print(), input(), etc.
- Module Functions : These functions are defined in specific modules. We can use them in our program after importing that module. For example, to use mathematical functions like sqrt(), ceil(), floor(), etc., we have to import the math module in our program.
- User Defined Functions : These functions are defined or created by the programmer for performing a specific task in a program.
Note: In this lesson, we will learn how to create and use a user-defined function in python.
Creating a User Defined Function
To create a user-defined function, we have to use the keyword def, as shown below.
Syntax of User Defined Function
def function_name(parameters):
statement 1
statement 2
...
Example (without parameters)
def message():
print('Hello I am learning how to create function in python.')
Example (with parameters)
def sum(a,b):
c=a+b
print('Sum of %d and %d is %d' %(a,b,c))
Note: Parameters are also called Arguments.
Calling a User Defined Function
To call a user-defined function, we have to call it by its name. See the complete example given below.
Example
def message():
print('Hello I am learning how to create function in python.')
def sum(a,b):
c=a+b
print('Sum of %d and %d is %d' %(a,b,c))
# main program
# calling the function message()
message()
# calling the function sum()
sum(10,20)
Output
Hello I am learning how to create function in python. Sum of 10 and 20 is 30
In the above program, we have declared two functions message() and sum(). The message() function does not accept any argument but displays a text on the screen whenever we call it. On the other hand, the sum() function accept two arguments and display their sum on the screen.
Flow of Execution of Function
The Flow of Execution of Function refers to the order in which statements are executed in the main program when any function is called. Let's see the execution of the above program using a diagram.
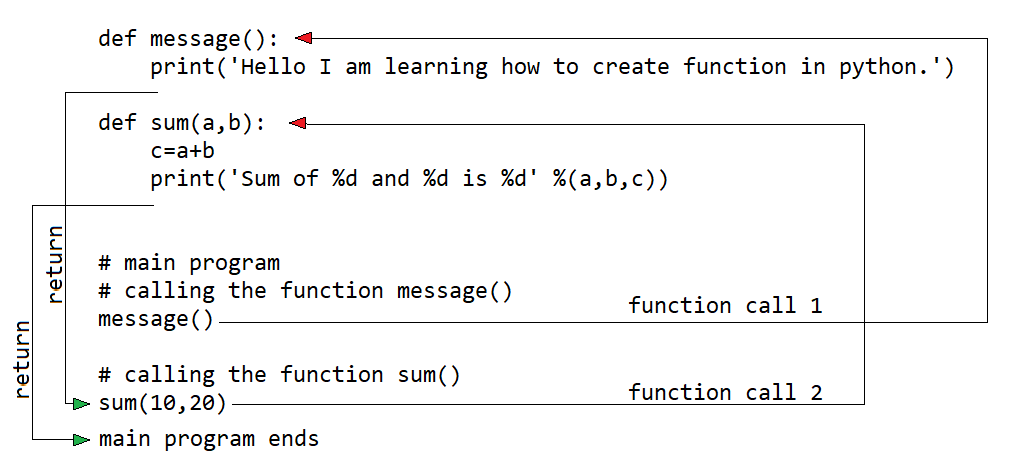
In the above diagram, we see that when the main program calls the function message(), the program flow goes to the body of the function message() and execute its codes. After that, it returns to the main program and then calls the second function sum() by sending it two arguments (10,20), the program flow goes to the body of the function sum(), and execute its code and again returns to the main program. At last, the main programs end.
Passing Different Types of Arguments in Function
We can pass different types of arguments in a function like an integer, float, string, list, object etc. Let's see some examples.
Example 1 (passing integers and float)
# a function defined to calculate simple interest
def simpleinterest(p,r,t):
si=(p*r*t)/100
print('Simple Interest =',si)
# main program
principal=int(input('Enter principal amount: '))
rate=float(input('Enter yearly rate %: '))
time=int(input('Enter time in year: '))
simpleinterest(principal,rate,time)
Output
Enter principal amount: 5000 Enter yearly rate %: 6.25 Enter time in year: 1 Simple Interest = 312.5
Example 2 (passing string)
# a function defined to count the total number of vowels in a string
def countvowels(s):
vc=0 # a varaible for counting vowels
for ch in s:
ch=ch.lower()
if ch=='a' or ch=='e' or ch=='i' or ch=='o' or ch=='u':
vc=vc+1
print('Total Vowels =',vc)
# main program
str=input('Enter a string: ')
countvowels(str)
Output
Enter a string: Dremendo Total Vowels = 3
Example 3 (passing list)
# a function defined to find the sum of the numbers in a list
def sum(data):
s=0 # a variable to calculate the sum of the numbers in a list
for n in data:
s=s+n
print('Sum of Numbers =',s)
# main program
a=list() # an empty list
print('Enter 5 number')
for i in range(0,5):
a.append(int(input()))
sum(a)
Output
Enter 5 number 5 8 2 4 6 Sum of Numbers = 25
Example 4 (passing object)
# As we know that functions are objects so we can pass it as an argument to another function
def uppercase(txt):
return txt.upper()
def lowercase(txt):
return txt.lower()
def display(func):
result = func('Hello Python')
return result
str1=display(uppercase)
print(str1)
str2=display(lowercase)
print(str2)
Output
HELLO PYTHON hello python
Returning Results from a Function
We can return one or more results or output from a function using the keyword return. The result can be an integer, float, string, list or any other type.
Example 1 (return one result)
# a function defined to return the cube of a number
def cube(n):
c=n*n*n
return c
# main program
x=int(input('Enter a number '))
print('Cube of %d = %d' %(x,cube(x)))
Output
Enter a number 5 Cube of 5 = 125
In the above example, we are returning a single output that is the cube of a number stored in variable c using the statement return c. The statement returns the value of variable c to the main program that prints the value on the screen.
Example 2 (return multiple results)
# a function defined to return the addition, subraction, multiplication and division of two given numbers
def calculate(a,b):
add=a+b
sub=a-b
mul=a*b
div=a/b
return add, sub, mul, div
# main program
print('Enter 2 numbers')
x=int(input())
y=int(input())
a,b,c,d=calculate(x,y)
print('Addition =',a)
print('Subtraction =',b)
print('Multiplication =',c)
print('Division =',d)
Output
Enter 2 numbers 5 2 Addition = 7 Subtraction = 3 Multiplication = 10 Division = 2.5
In the above example, we are returning multiple outputs using the statement return add, sub, mul, div. The statement returns the value of variables add, sub, mul and div to the main program that gets stored in the variable a, b, c and d respectively.
Formal and Actual Arguments
The arguments or parameters used during function declaration within the round brackets ( ) are known as Formal Arguments. Whereas the arguments or parameters that are used when providing input to the function from the main program are known as Actual Arguments. Let's see an example for more understanding.
Example
# a function defined to calculate simple interest
def simpleinterest(p,r,t):
si=(p*r*t)/100
print('Simple Interest =',si)
# main program
principal=int(input('Enter principal amount: '))
rate=float(input('Enter yearly rate %: '))
time=int(input('Enter time in year: '))
simpleinterest(principal,rate,time)
In the above program, variable p, r and t in function simpleinterest() are used to receive inputs from the main program, so these variables are known as Formal Arguments.
The variables principal, rate and time used in the main program to provide inputs to the function simpleinterest() are known as Actual Arguments because they provide the actual inputs to the function simpleinterest().
Actual Arguments further can be of four types, and they are:
- Positional Argument
- Keyword or Named Argument
- Default Argument
- Variable Length Arguments
Let's discuss each of them one by one in more detail.
Positional Argument
Passing the number of arguments to a function in the order they are declared in the function is known as Positional Argument. The total numbers of positional arguments must match with total numbers of formal arguments required by the function for processing the task. Let's take an example.
Example
# a function defined to return the sum of three numbers
def sum(a,b,c):
s=a+b+c
return s
# main program
print('Enter 3 numbers')
x=int(input())
y=int(input())
z=int(input())
s=sum(x,y,z)
print('Sum =',s)
In the above program, there are three formal arguments a, b and c declared in the function sum(). Now, when we send the values to these variables using the actual arguments x, y and z, the value of x is received by a, the value of y is received by b and the value of z is received by c. This way of passing arguments to the function is known as Positional Arguments.
Keyword or Named Argument
If we want to pass arguments to a function without maintaining its order, then this can be done by a process called Keyword or Named Argument. In this process, we send the value to the function with its variable names defined in the function. Consider the example of the function simpleinterest().
Example
# a function defined to calculate simple interest
def simpleinterest(p,r,t):
si=(p*r*t)/100
print('Simple Interest =',si)
# main program
principal=int(input('Enter principal amount: '))
rate=float(input('Enter yearly rate %: '))
time=int(input('Enter time in year: '))
simpleinterest(r=rate,t=time,p=principal)
In the above program, we are sending the values to the function simpleinterest() without maintaining the order of the variables declared in the function. Instead, we are sending the values to the function using the variable names defined in it.
Default Argument
Default Argument is useful when we want to set a default value to one or more variables defined in a function so that when no value is passed to these variables from the main program. The function will use the default value of these variables for processing the task. See the example given below.
Example
# a function defined to calculate simple interest
def simpleinterest(p,r=5.3,t=1):
si=(p*r*t)/100
print('Simple Interest =',si)
# main program
principal=int(input('Enter principal amount: '))
time=int(input('Enter time in year: '))
simpleinterest(p=principal,t=time)
Output
Enter principal amount: 5000 Enter time in year: 2 Simple Interest = 530.0
In the above program, we are sending only the values for p and t to the function simpleinterest() using the Keyword Arguments except for the value of r. In this case, the function will use the default value of r that is 5.3 to calculate the simple interest.
Variable Length Arguments
Variable Length Arguments are useful in a situation when we don't know how many arguments are needed to be defined in the function to process a task. For example, if we want to find the sum of numbers using a function but don't know how many arguments we need, in this case, we use Variable Length Arguments.
Example
# a function defined to calculate the sum of numbers
def sum(*num):
s=0
for x in num:
s=s+x
print('Sum =',s)
# main program
print('Sum of 2 numbers 10,20')
sum(10,20)
print('Sum of 3 numbers 10,20,50')
sum(10,20,50)
print('Sum of 4 numbers 10,20,40,90')
sum(10,20,40,90)
Output
Sum of 2 numbers 10,20 Sum = 30 Sum of 3 numbers 10,20,50 Sum = 80 Sum of 4 numbers 10,20,40,90 Sum = 160
In the above program, we have declared a Variable Length Argument in the function sum() using an asterisk symbol * in front of a variable name num. Now the variable *num can receive multiple numbers in the function. To read each number from the variable *num, we have to run a for loop as shown above.
Nested or Inner Function
Python allows us to create functions inside another function, and this is known as the Nested Function or Inner Function. Nested functions are useful when we want to process some task using the helper function inside the parent function.
Example
A Python program to store 10 numbers in a list and find the sum all the prime numbers present in the list using function.
# a function defined to calculate the sum of all prime numbers in a list
def primesum(num):
sum=0
# inner function to check if a number is prime or not
def isprime(n):
f=0 # a variable for counting factors of the given number
for i in range(1,n+1):
if n%i==0:
f=f+1
if f==2: # a prime number has only 2 factors that is 1 and itself
return True
else:
return False
for x in num:
if isprime(x)==True:
sum=sum+x
return sum
# main program
n=[15,7,3,56,9,5,17,24,31,11]
print('List: ',n)
s=primesum(n)
print('Sum of all prime numbers =',s)
Output
List: [15, 7, 3, 56, 9, 5, 17, 24, 31, 11] Sum of all prime numbers = 74
Scope of Variables
The Scope of Variables means the region or area in a program in which we can access the defined variables.
The scope of a variable depends upon its declaration in a program. If the variable is declared outside the function or in global scope, then it can be accessed from anywhere in the program. If it is declared inside the function or in local scope, then it can only be accessed from within the region in which it is declared. Let's see an example of global and local variables in a program.
Example
x='global' # declared a global variable x
def message():
x='local' # declared a local variable x
print(x) # printing the value of local variable x
# main program
message()
print(x) # printing the value of global variable x
Output
local global
In the above program, we have declared a global variable x outside and a local variable x inside the function message(). When we are printing the value of x inside the function message(), then we are accessing the local variable x not the global variable x. On the other hand, when we are printing the value of x inside the main program, then we are accessing the global variable x.
To access the global variable x inside the function message(), we have to use the keyword global. See the example given below.
Example
x='global' # declared a global variable x
def message():
global x # redeclare the global variable x with the global keyword to access or change it value
print(x) # printing the value of global variable x
x='python' # now we can access or change the value of the global variable x
print(x) # printing the current value of global variable x
# main program
message()
Output
global python
In the above example, to access the global variable x in the function message(), we have to redeclare it with the global keyword. After that, we can access or change the value of the global variable x inside the function message(), as shown above.
Nonlocal Keyword in Function
The nonlocal keyword is used in the inner function to access or change the value of a variable declared in the local scope of the outer function.
Example
def message():
x='local' # declared a local variable x in outer function message()
def display():
nonlocal x # redeclare variable x of outer function with the nonlocal keyword to access or change its value
x='python' # now we can access or change the value of the local variable x of outer function
print(x) # printing the local variable x of outer function message()
display()
print(x) # printing the local variable x of outer function message() after executing the display()
# main program
message()
Output
local python
In the above program, to access the local variable x of the outer function message() inside the function display(), we have to redeclare it with the nonlocal keyword. After that, we can access or change the value of the local variable x inside the function display(), as shown above.
Test Your Knowledge
Attempt the practical questions to check if the lesson is adequately clear to you.
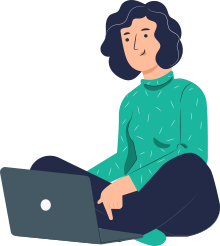